Snap Interactions
https://developer.oculus.com/documentation/unity/unity-isdk-snap-interaction/
Snap interactions allow items to automatically snap to poses in the environment.
스냅 상호 작용을 사용하면 항목이 환경의 포즈에 자동으로 스냅될 수 있습니다.
The DistanceGrabExample scene uses them to simply have the items snap back to their original position when not in use,
but it is possible to create custom behaviors so the objects snap to inventories, cells in a board game, slots around the user body, etc.
DistanceGrabExample 씬은 사용하지 않을 때 단순히 아이템을 원래 위치로 되돌리기 위해 이를 사용하지만,
오브젝트가 인벤토리, 보드 게임의 셀, 사용자 몸 주위의 슬롯 등에 스냅되도록 커스텀 동작을 만들 수 있습니다.
SnapInteractor
The SnapInteractor is the Interactor class for this interaction.
SnapInteractor는 이 상호 작용에 대한 Interactor 클래스입니다.
This component is placed in the item that we want to be able to snap, e.g. a chess piece,
and will take care of detecting nearby SnapInteractables and move the item towards the best available pose provided by them.
이 컴포넌트는 스냅할 수 있는 아이템(예: 체스 말)에 배치되며,
근처의 SnapInteractables를 감지하고 아이템이 제공하는 최상의 포즈로 아이템을 이동합니다.
When the Optional TimeOutInteractable and TimeOut are provided,
the Interactor will automatically move to said interactable after TimeOut seconds
if the Grabbable is not being Selected or Hovered by any other interactor.
선택적 TimeOutInteractable 및 TimeOut이 제공되면 다른 인터랙터가
Grabbable을 선택하거나 가리키지 않는 경우 인터랙터는 TimeOut(초) 후에 자동으로 해당 인터랙터로 이동합니다.
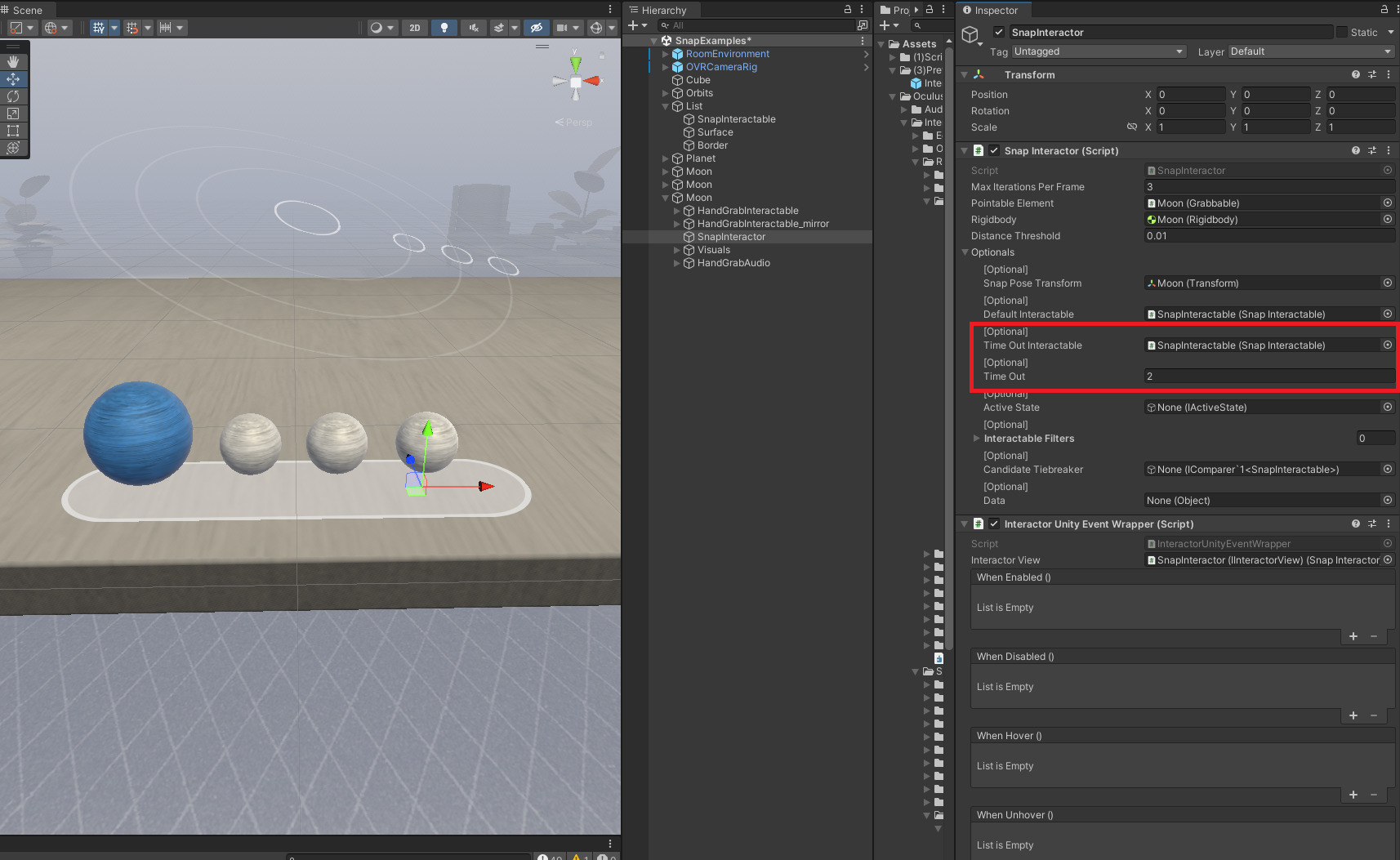
SnapInteractable
The SnapInteractable component is the Interactable class for this interaction.
SnapInteractable 구성 요소는 이 상호 작용에 대한 Interactable 클래스입니다.
When used as-is it provides a single pose in space to which the interactors can snap too, but optionally it can be enhanced in several ways.
있는 그대로 사용하면 상호 작용자가 스냅할 수 있는 공간에서 단일 포즈를 제공하지만 선택적으로 여러 가지 방법으로 향상시킬 수 있습니다.
Trigger collider
when a Trigger volume is present in the same gameobject,
Interactors entering the volume will be tracked and automatically snap to the interactable pos as soon as their state becomes equal to Normal.
트리거 볼륨이 동일한 게임 오브젝트에 있는 경우 볼륨에 진입하는 인터랙터가 추적되고
상태가 Normal이 되는 즉시 상호 작용 가능한 위치에 자동으로 스냅됩니다.
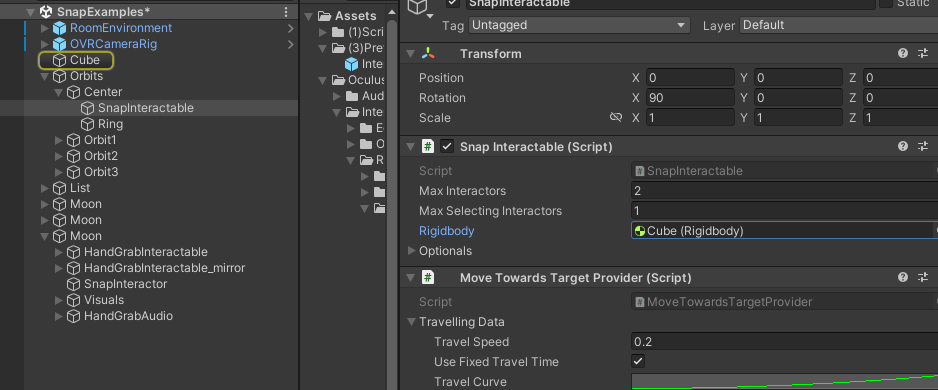
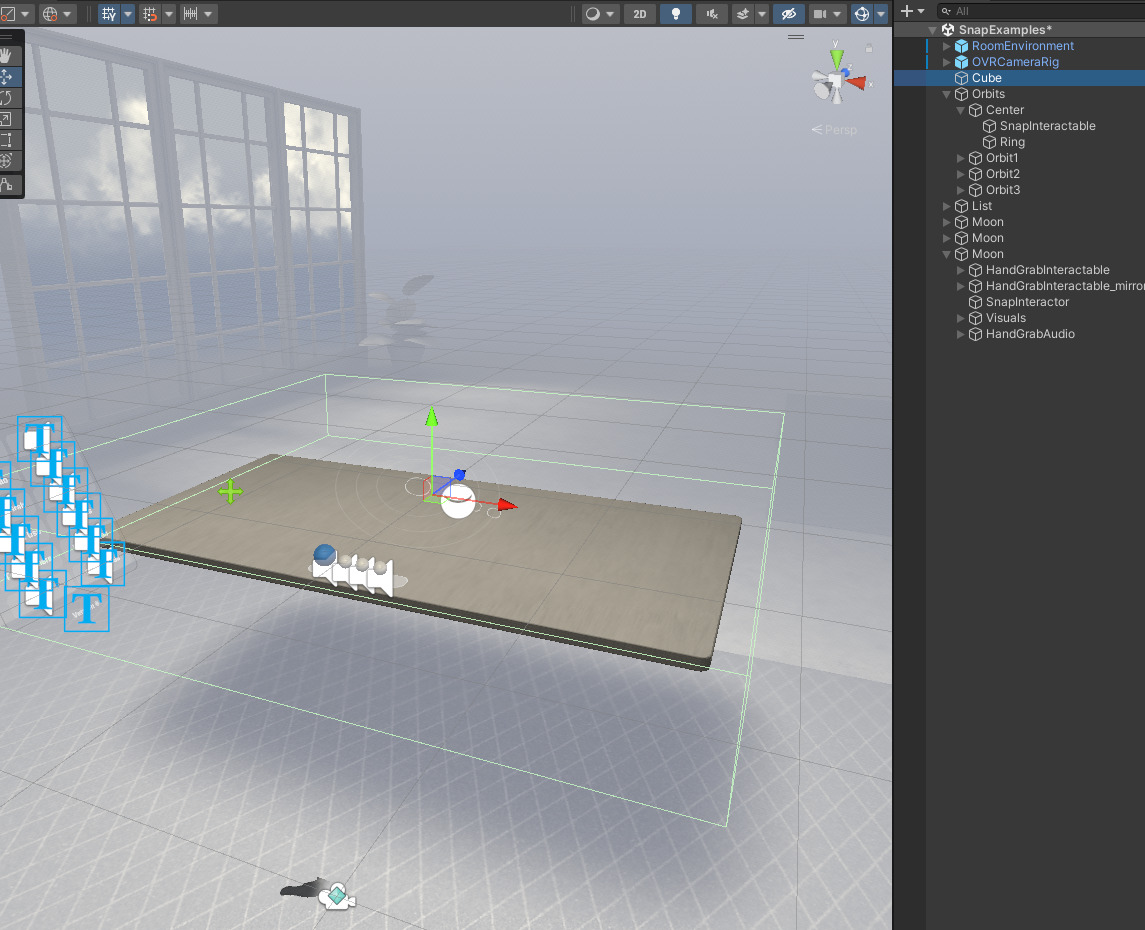
MovementProvider
by referencing a MovementProvider the method for snapping can be fully customized.
MovementProvider를 참조하여 스냅 메서드를 완전히 사용자 지정할 수 있습니다.
By default the interactor will follow the slot at a fixed speed, but following the different MovementProvider it could trace a curve, add easing, etc.
기본적으로 인터랙터는 고정된 속도로 슬롯을 따라가지만 다른 MovementProvider를 따라 커브를 추적하고 부드럽게를 추가하는 등의 작업을 수행할 수 있습니다.
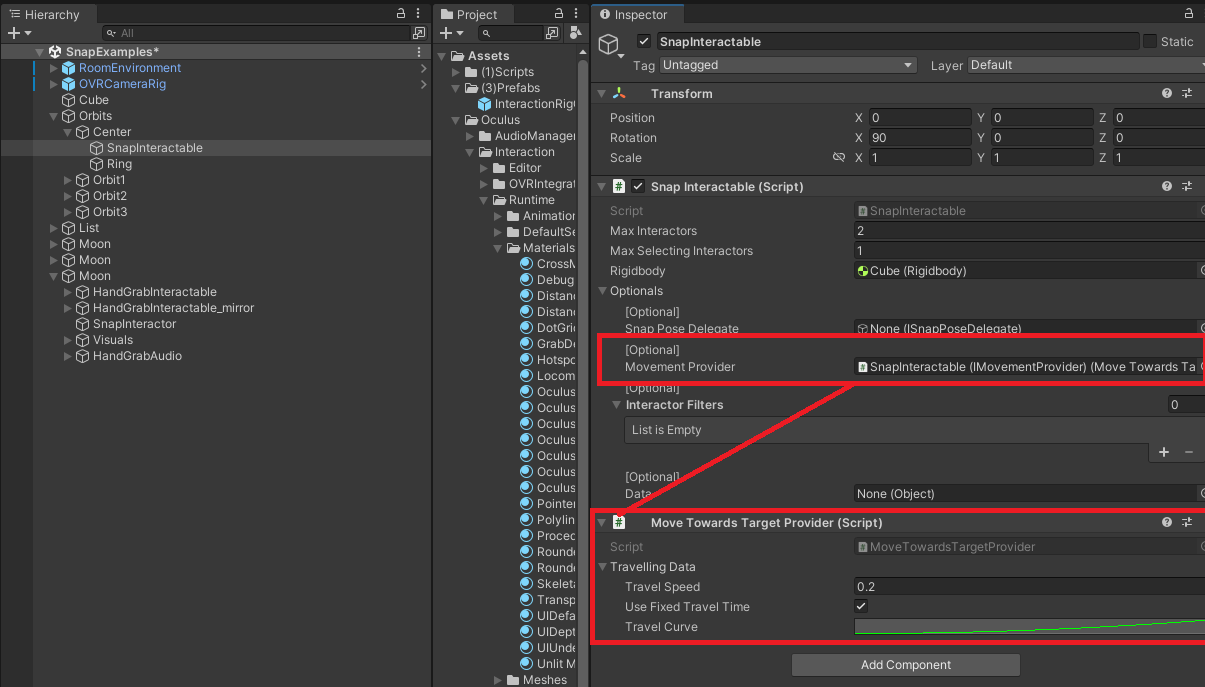
SnapPosesProvider
the ISnapPoseProvider interface allows creating multiple poses within a SnapInteractable.
ISnapPoseProvider 인터페이스를 사용하면 SnapInteractable 내에서 여러 포즈를 만들 수 있습니다.
Implementing and providing this interface is useful for using a single SnapInteractable for all the cells within a board in a board game,
or to define all the different slots in an inventory system, allowing to even move snapped items when hovering new interactors to make room.
이 인터페이스를 구현하고 제공하면 보드 게임의 보드 내의 모든 셀에 대해 단일 SnapInteractable을 사용하거나 인벤토리 시스템에서 모든 다른 슬롯을 정의하는 데 유용하며,
공간을 만들기 위해 새 상호 작용기를 마우스로 가리킬 때 스냅된 항목을 이동할 수도 있습니다.
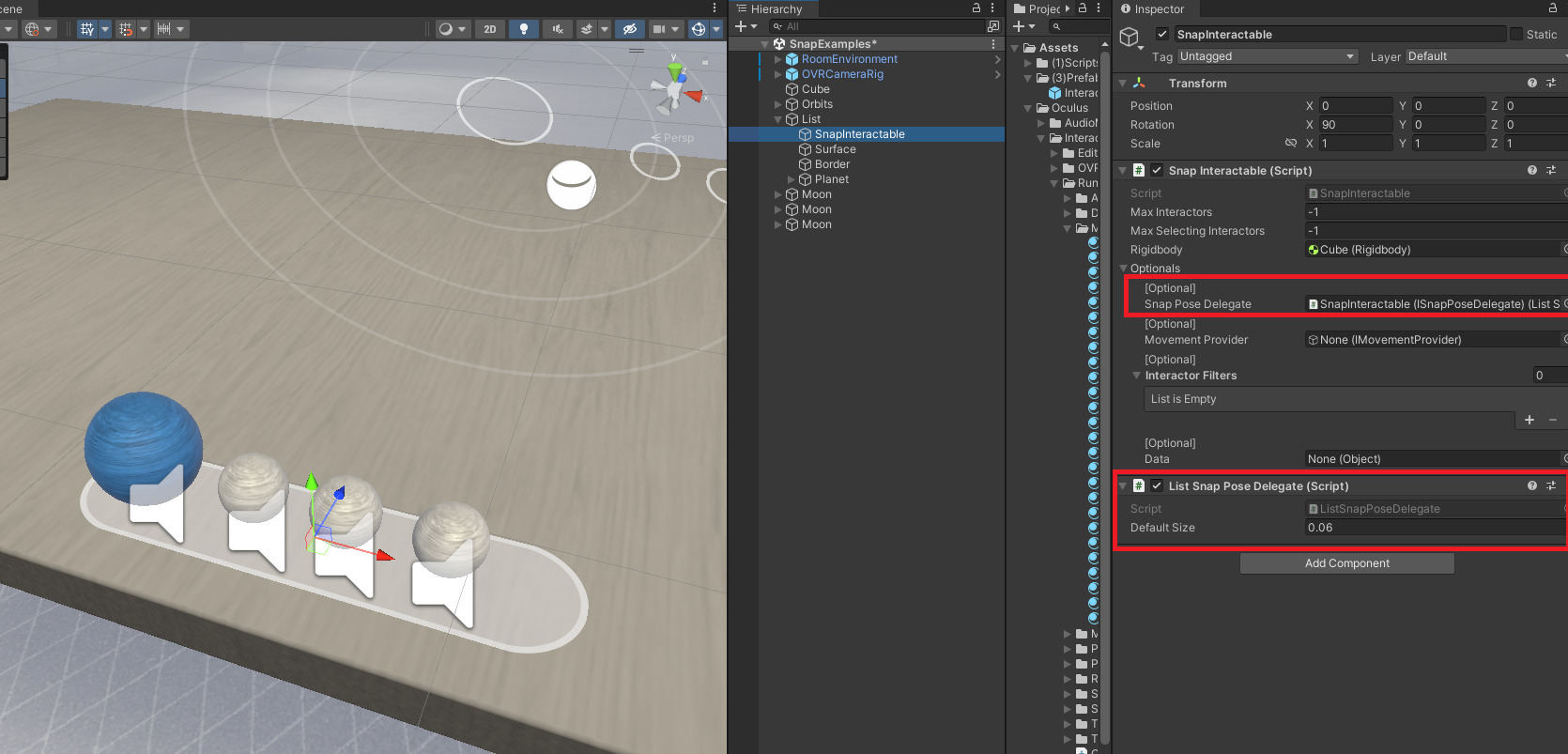
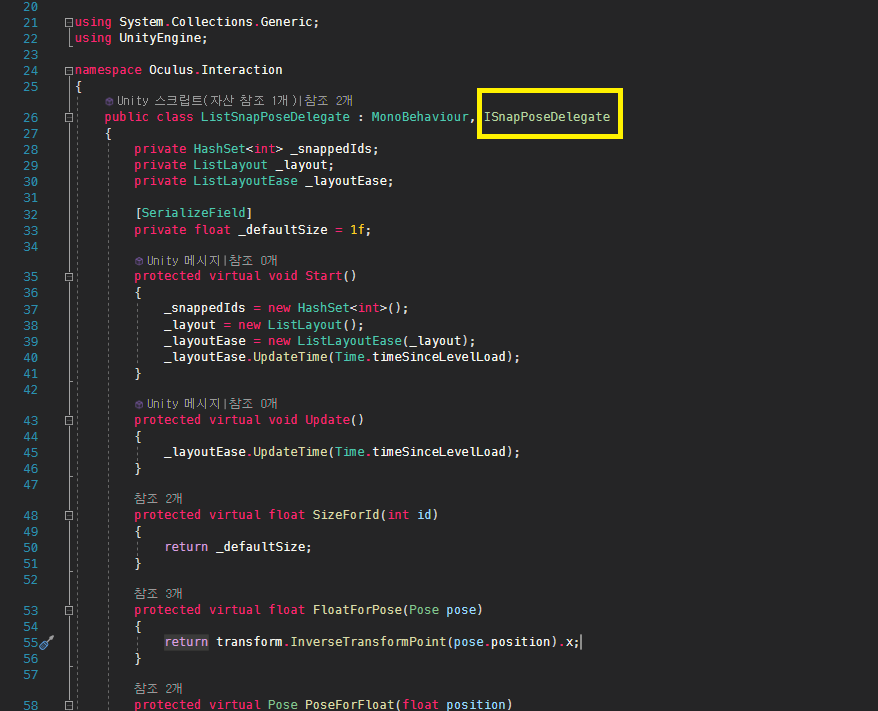
using UnityEngine; namespace Oculus.Interaction { /// <summary> /// SnapPoseDelegate는 맞춤형 SnapPose 로직을 제공하는 데 사용할 수 있습니다 /// 추적되거나 스냅되는 요소 집합이 주어집니다. /// </summary> public interface ISnapPoseDelegate { /// <summary> /// 새 요소가 추적 중임을 나타냅니다. /// </summary> /// <param name="id">추적할 요소 ID입니다.</param> /// <param name="params">요소의 포즈입니다.</param> void TrackElement(int id, Pose p); /// <summary> /// 요소가 더 이상 추적되지 않음을 나타냅니다. /// </summary> /// <param name="id">추적을 중지할 요소 ID입니다.</param> void UntrackElement(int id); /// <summary> /// 추적된 요소가 스냅되어야 함을 나타냅니다. /// </summary> /// <param name="id">스냅할 요소 ID입니다.</param> /// <param name="params">요소의 포즈입니다.</param> void SnapElement(int id, Pose pose); /// <summary> /// 요소가 더 이상 스냅되지 않아야 함을 나타냅니다. /// </summary> /// <param name="id">스냅을 중지할 요소 ID입니다.</param> void UnsnapElement(int id); /// <summary> /// 추적된 요소 포즈가 업데이트되었음을 나타냅니다. /// </summary> /// <param name="id">요소 ID.</param> /// <param name="params">새로운 요소 포즈.</param> void MoveTrackedElement(int id, Pose p); /// <summary> /// 쿼리된 요소 ID에 대한 대상 스냅 포즈입니다. /// </summary> /// <param name="id">요소 ID.</param> /// <param name="params">타깃 포즈.</param> /// <returns>요소에 스냅할 포즈가 있으면 참입니다.</returns> bool SnapPoseForElement(int id, Pose pose, out Pose result); } }