ECS – Entity concepts
https://docs.unity3d.com/Packages/com.unity.entities@1.3/manual/concepts-entities.html
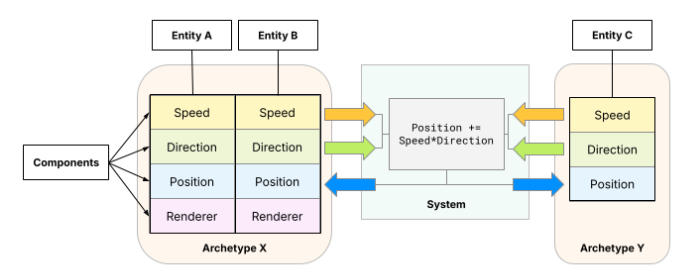
1. Entity란?
- 프로그램 내에서 특정 개체(캐릭터, 이펙트, UI 요소 등)를 나타내는 단순한 ID 역할
- 기존
GameObject
와 유사하지만, 코드나 데이터를 포함하지 않고 컴포넌트들의 조합을 통해 기능을 정의 - 개별 Entity는 World에 속하며,
EntityManager
가 이를 관리
2. EntityManager (Entity 관리)
EntityManager
는 Entity를 생성, 삭제, 수정하는 다양한 메서드를 제공
메서드 | 설명 |
---|---|
CreateEntity() | 새로운 Entity 생성 |
Instantiate(Entity e) | 기존 Entity를 복사하여 새로운 Entity 생성 |
DestroyEntity(Entity e) | 특정 Entity 삭제 |
AddComponent<T>(Entity e) | Entity에 특정 컴포넌트 추가 |
RemoveComponent<T>(Entity e) | Entity에서 특정 컴포넌트 제거 |
GetComponent<T>(Entity e) | Entity의 특정 컴포넌트 값 가져오기 |
SetComponent<T>(Entity e, T data) | Entity의 특정 컴포넌트 값 변경 |
Entity의 생성/삭제는 구조적 변경(Structural Change)을 일으켜 성능에 영향을 줄 수 있음
예시
- Entity 생성 & 삭제
using Unity.Entities; using Unity.Collections; using UnityEngine; public class EntityManagerExample : MonoBehaviour { private EntityManager entityManager; private Entity entity; private void Start() { // 현재 월드의 EntityManager 가져오기 entityManager = World.DefaultGameObjectInjectionWorld.EntityManager; // 새로운 Entity 생성 (빈 상태) entity = entityManager.CreateEntity(); Debug.Log($"Entity 생성됨: {entity.Index}"); // Entity 삭제 entityManager.DestroyEntity(entity); Debug.Log("Entity 삭제됨"); } }
- 컴포넌트 추가 & 제거
using Unity.Entities; using UnityEngine; // [IComponentData]를 상속받은 간단한 컴포넌트 public struct Health : IComponentData { public int Value; } public class AddRemoveComponentExample : MonoBehaviour { private EntityManager entityManager; private Entity entity; private void Start() { entityManager = World.DefaultGameObjectInjectionWorld.EntityManager; entity = entityManager.CreateEntity(); // 컴포넌트 추가 entityManager.AddComponentData(entity, new Health { Value = 100 }); Debug.Log("Health 컴포넌트 추가됨"); // 컴포넌트 제거 entityManager.RemoveComponent<Health>(entity); Debug.Log("Health 컴포넌트 제거됨"); } }
- 컴포넌트 값 읽기(Get) & 수정(Set)
using Unity.Entities; using UnityEngine; public class GetSetComponentExample : MonoBehaviour { private EntityManager entityManager; private Entity entity; private void Start() { entityManager = World.DefaultGameObjectInjectionWorld.EntityManager; entity = entityManager.CreateEntity(); // Health 컴포넌트 추가 (초기 값 100) entityManager.AddComponentData(entity, new Health { Value = 100 }); Debug.Log($"초기 체력: {entityManager.GetComponentData<Health>(entity).Value}"); // Health 값 변경 entityManager.SetComponentData(entity, new Health { Value = 50 }); Debug.Log($"변경된 체력: {entityManager.GetComponentData<Health>(entity).Value}"); } }
3. Entity와 Archetype (아키타입)
- Entity 자체에는 타입이 없으며, Entity를 구성하는 컴포넌트 조합이 그 특성을 결정
- 같은 컴포넌트 조합을 가진 Entity들은 동일한 아키타입(Archetype)을 공유
EntityManager
는 현재 존재하는 Entity들의 고유한 컴포넌트 조합(Archetype)을 추적
Archetype을 활용하면 ECS 데이터 구조를 최적화하고 성능을 향상시킬 수 있음
4. Entity 관리 구조
- 모든 Entity는 World 내에서 관리됨
EntityManager
는 Entity의 생성, 삭제 및 컴포넌트 조작을 담당- Entity는 컴포넌트들의 조합(Archetype)으로 정의됨
요약
- Entity는 단순한 ID 역할을 하며, 실제 기능은 컴포넌트(Component)를 통해 정의됨
- EntityManager를 사용하여 Entity를 생성(Create), 삭제(Destroy), 수정(Set, Get) 가능
- Entity는 컴포넌트 조합(Archetype) 단위로 그룹화되며, 동일한 Archetype을 가진 Entity 는 메모리에서 최적화됨
- Entity의 생성/삭제는 구조적 변경(Structural Change)을 일으켜 성능에 영향을 줄 수 있음