Unity Version : 2021.3.5f1
https://docs.unity3d.com/kr/2019.4/Manual/script-AnimationWindowEvent.html <- 공식 문서
https://assetstore.unity.com/packages/3d/characters/humanoids/barbarian-warrior-75519 <- 테스트 에셋
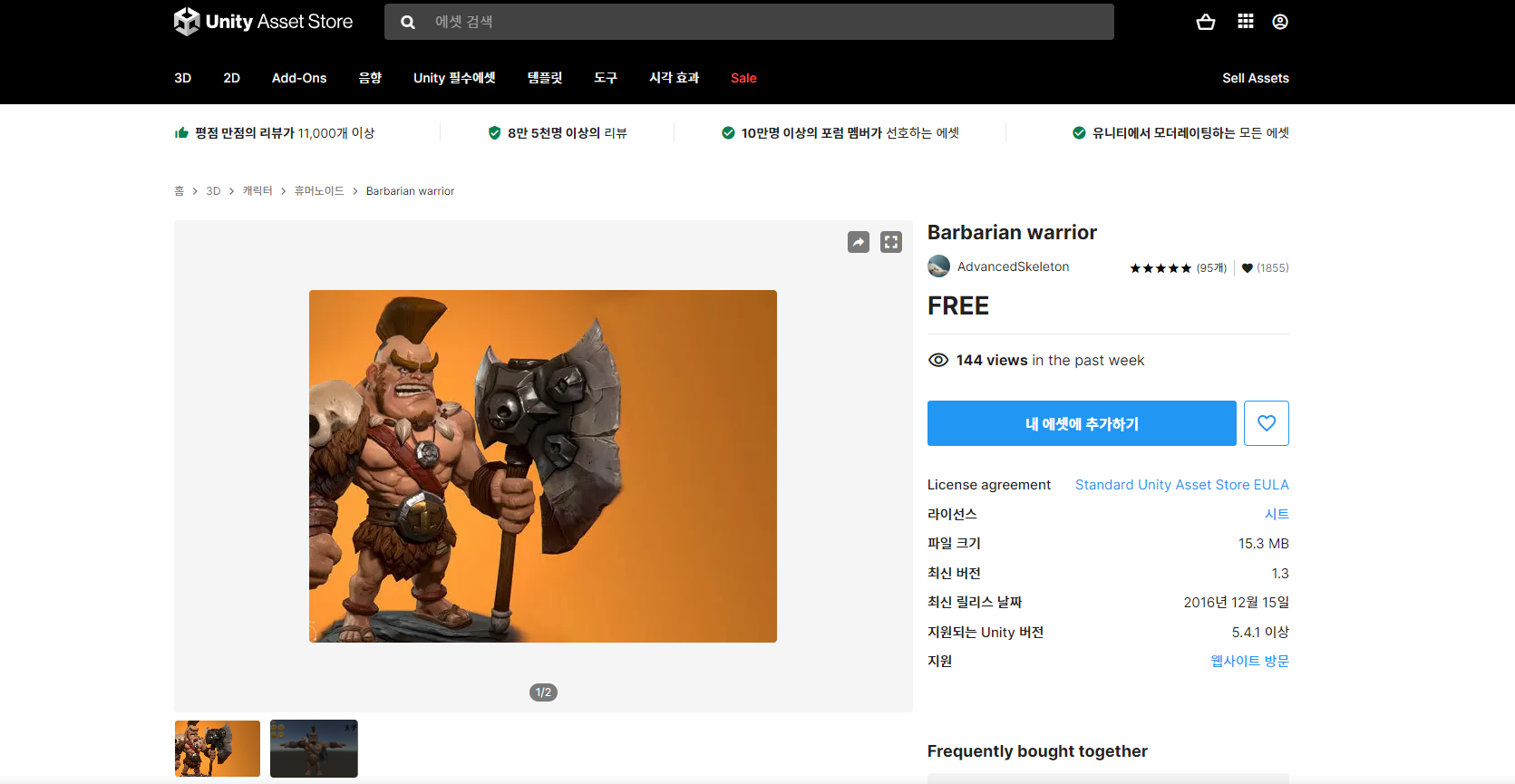
스크립트로 애니메이션 이벤트 추가
바바리안 모델은 애니메이션 컨트롤러가 없다. (직접 만들어주자)
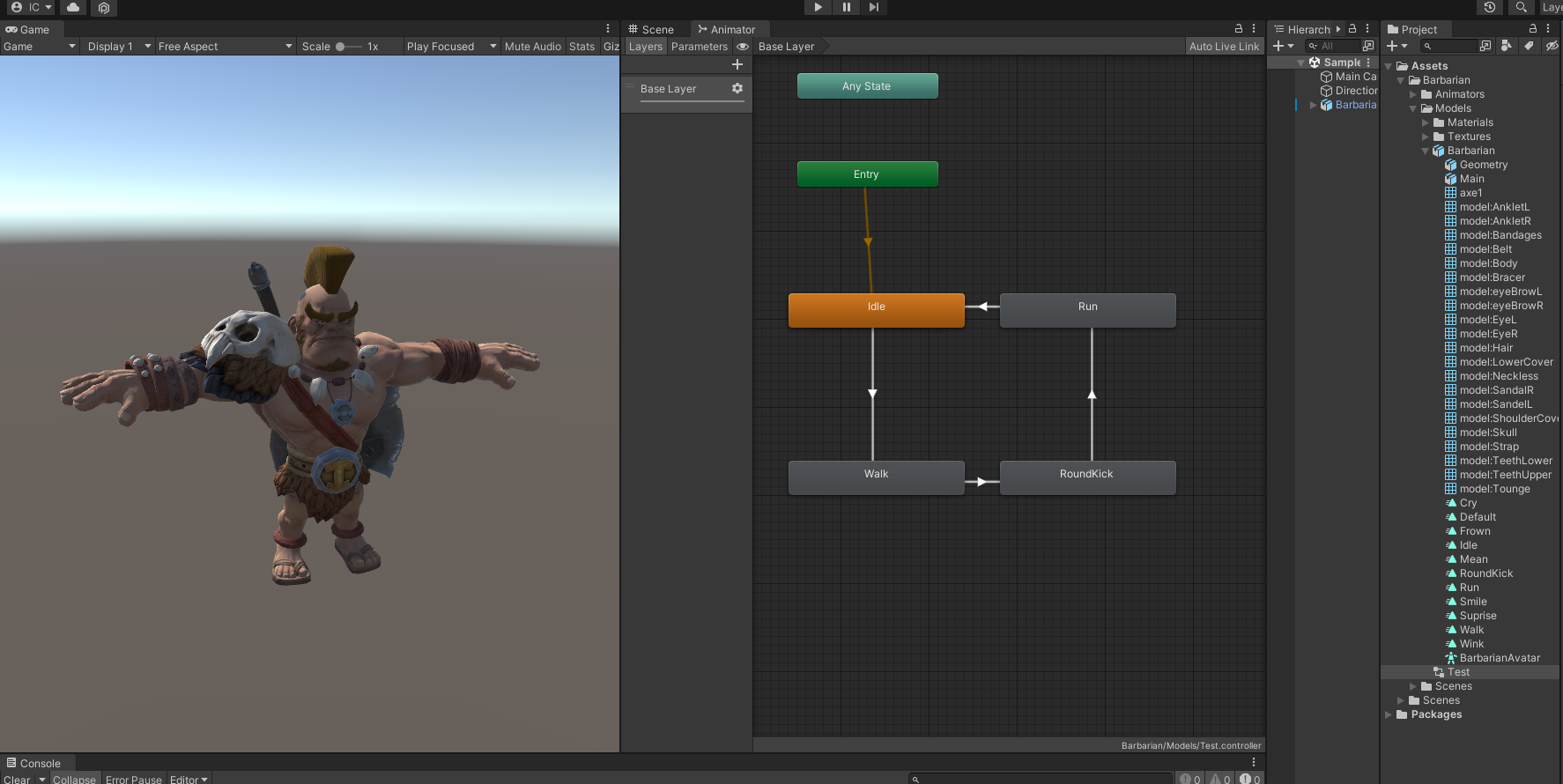
각 Idle, Walk의 애니메이션이 끝나면 이벤트를 실행 (Run과 RoundKick은 X )
using UnityEngine; public class Test : MonoBehaviour { private Animator ani; public AnimationClip Idle; public AnimationClip Walk; private void Awake() { this.ani = this.gameObject.GetComponent<Animator>(); this.Idle.AddEvent(new AnimationEvent() { time = Idle.length, // 애니메이션 클립의 길이를 받아서 이벤트 추가 functionName = "FunctionIdle" // 함수의 이름과 같아야한다. }); this.Walk.AddEvent(new AnimationEvent() { time = Walk.length, functionName = "FunctionWalk" }); } private void FunctionIdle() { Debug.Log("Idle 실행"); } private void FunctionWalk() { Debug.Log("Walk 실행"); } }
애니메이션에서 직접 이벤트 추가
바바리안 애니메이션은 Read only라서 수정이 불가능하다. (이벤트 추가 X)
새로운 애니메이션을 만들고 Run을 복붙하면 수정가능하다.
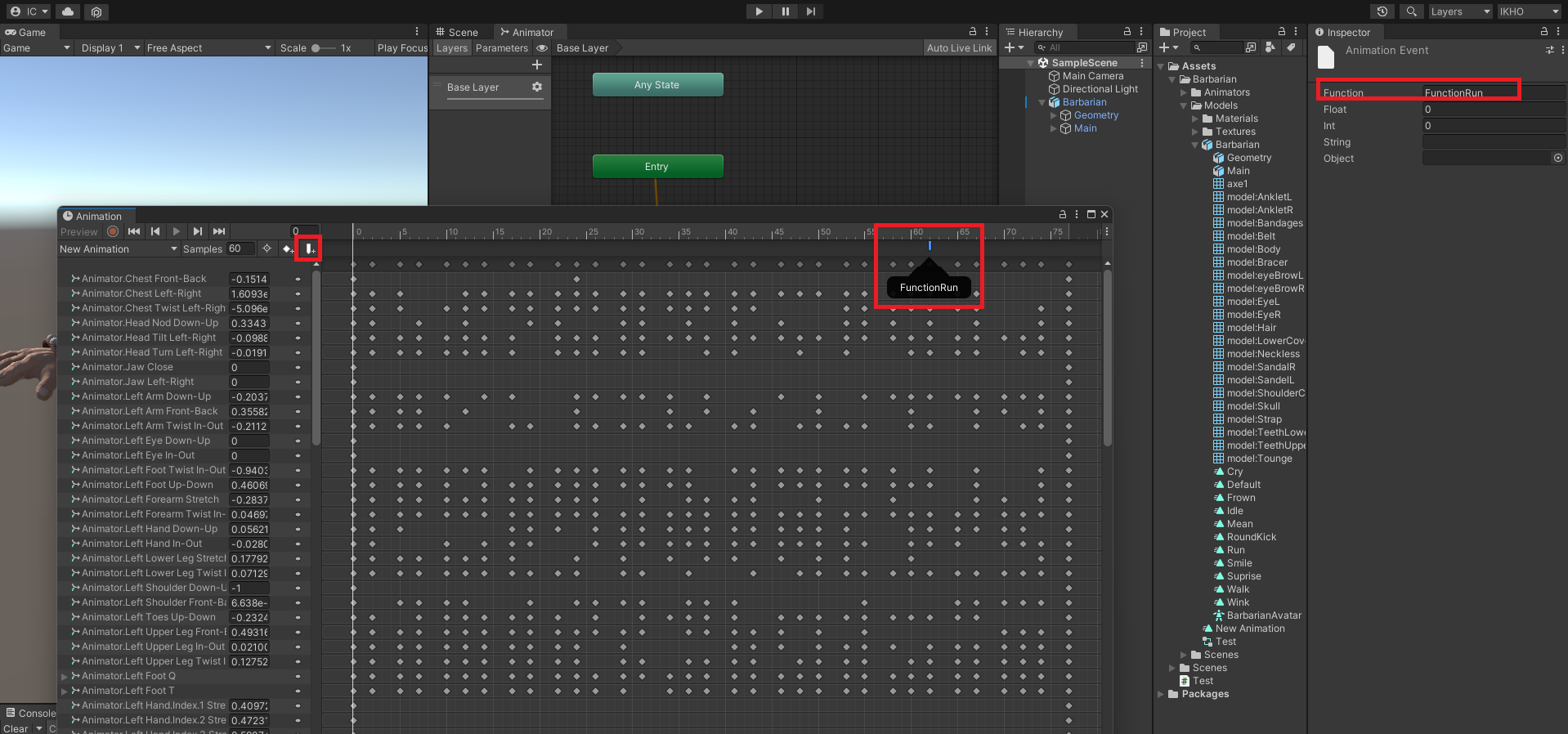
using UnityEngine; public class Test : MonoBehaviour { private Animator ani; public AnimationClip Idle; public AnimationClip Walk; private void Awake() { this.ani = this.gameObject.GetComponent<Animator>(); this.Idle.AddEvent(new AnimationEvent() { time = Idle.length, functionName = "FunctionIdle" }); this.Walk.AddEvent(new AnimationEvent() { time = Walk.length, functionName = "FunctionWalk" }); } private void FunctionIdle() { Debug.Log("Idle 실행"); } private void FunctionWalk() { Debug.Log("Walk 실행"); } private void FunctionRun() { Debug.Log("Run 실행"); } }
애니메이션 이벤트 파라미터 사용하기
https://forum.unity.com/threads/how-to-use-multiple-param-in-animation-event.364138/ <- 참고
using UnityEngine; public class Test : MonoBehaviour { private Animator ani; public AnimationClip Idle; private void Awake() { this.ani = this.gameObject.GetComponent<Animator>(); this.Idle.AddEvent(new AnimationEvent() { time = Idle.length, intParameter = 100, stringParameter = "바보", functionName = "FunctionIdle" }) ; } private void FunctionIdle(AnimationEvent myEvent) { Debug.Log("Idle 실행"); Debug.Log(myEvent.intParameter); // 파라미터 사용 Debug.Log(myEvent.stringParameter); // 파라미터 사용 } private void FunctioasdnRun() { Debug.Log("Run 실행"); } }
참고
using System; using System.Runtime.InteropServices; using UnityEngine.Scripting; namespace UnityEngine { // // 요약: // AnimationEvent lets you call a script function similar to SendMessage as part // of playing back an animation. [Serializable] [StructLayout(LayoutKind.Sequential)] [RequiredByNativeCode] public sealed class AnimationEvent { internal float m_Time; internal string m_FunctionName; internal string m_StringParameter; internal Object m_ObjectReferenceParameter; internal float m_FloatParameter; internal int m_IntParameter; internal int m_MessageOptions; internal AnimationEventSource m_Source; internal AnimationState m_StateSender; internal AnimatorStateInfo m_AnimatorStateInfo; internal AnimatorClipInfo m_AnimatorClipInfo; [Obsolete("Use stringParameter instead")] public string data { get { return m_StringParameter; } set { m_StringParameter = value; } } // // 요약: // String parameter that is stored in the event and will be sent to the function. public string stringParameter { get { return m_StringParameter; } set { m_StringParameter = value; } } // // 요약: // Float parameter that is stored in the event and will be sent to the function. public float floatParameter { get { return m_FloatParameter; } set { m_FloatParameter = value; } } // // 요약: // Int parameter that is stored in the event and will be sent to the function. public int intParameter { get { return m_IntParameter; } set { m_IntParameter = value; } } // // 요약: // Object reference parameter that is stored in the event and will be sent to the // function. public Object objectReferenceParameter { get { return m_ObjectReferenceParameter; } set { m_ObjectReferenceParameter = value; } } // // 요약: // The name of the function that will be called. public string functionName { get { return m_FunctionName; } set { m_FunctionName = value; } } // // 요약: // The time at which the event will be fired off. public float time { get { return m_Time; } set { m_Time = value; } } // // 요약: // Function call options. public SendMessageOptions messageOptions { get { return (SendMessageOptions)m_MessageOptions; } set { m_MessageOptions = (int)value; } } // // 요약: // Returns true if this Animation event has been fired by an Animation component. public bool isFiredByLegacy => m_Source == AnimationEventSource.Legacy; // // 요약: // Returns true if this Animation event has been fired by an Animator component. public bool isFiredByAnimator => m_Source == AnimationEventSource.Animator; // // 요약: // The animation state that fired this event (Read Only). public AnimationState animationState { get { if (!isFiredByLegacy) { Debug.LogError("AnimationEvent was not fired by Animation component, you shouldn't use AnimationEvent.animationState"); } return m_StateSender; } } // // 요약: // The animator state info related to this event (Read Only). public AnimatorStateInfo animatorStateInfo { get { if (!isFiredByAnimator) { Debug.LogError("AnimationEvent was not fired by Animator component, you shouldn't use AnimationEvent.animatorStateInfo"); } return m_AnimatorStateInfo; } } // // 요약: // The animator clip info related to this event (Read Only). public AnimatorClipInfo animatorClipInfo { get { if (!isFiredByAnimator) { Debug.LogError("AnimationEvent was not fired by Animator component, you shouldn't use AnimationEvent.animatorClipInfo"); } return m_AnimatorClipInfo; } } // // 요약: // Creates a new animation event. public AnimationEvent() { m_Time = 0f; m_FunctionName = ""; m_StringParameter = ""; m_ObjectReferenceParameter = null; m_FloatParameter = 0f; m_IntParameter = 0; m_MessageOptions = 0; m_Source = AnimationEventSource.NoSource; m_StateSender = null; } internal int GetHash() { int num = 0; num = functionName.GetHashCode(); return 33 * num + time.GetHashCode(); } } }