허술한 도서 관리 프로그램
국비교육 프로그래밍을 들으면서 C#과 winform을 잘 몰랐을 때 만든 아주 허술한 프로그램입니다.
이 당시에 프로그래밍이란 걸 배운 지 1개월 약간 넘은 시기라서 만드는 데 5시간 넘게 걸린 것 같습니다.
Winform을 이용한 원격 수업 과제를 제출해야 해서 아주 단순한 기능 구현만 했습니다.
코딩을 할때 정렬 부분을 노가다로 처리한 것 / 구조 등 여러가지 많이 아쉽네요.
초보자가 작성한 코드라서 참고할 만한 부분이 있으지는 모르겠지만 필요하시면 쓰셔도 됩니다.
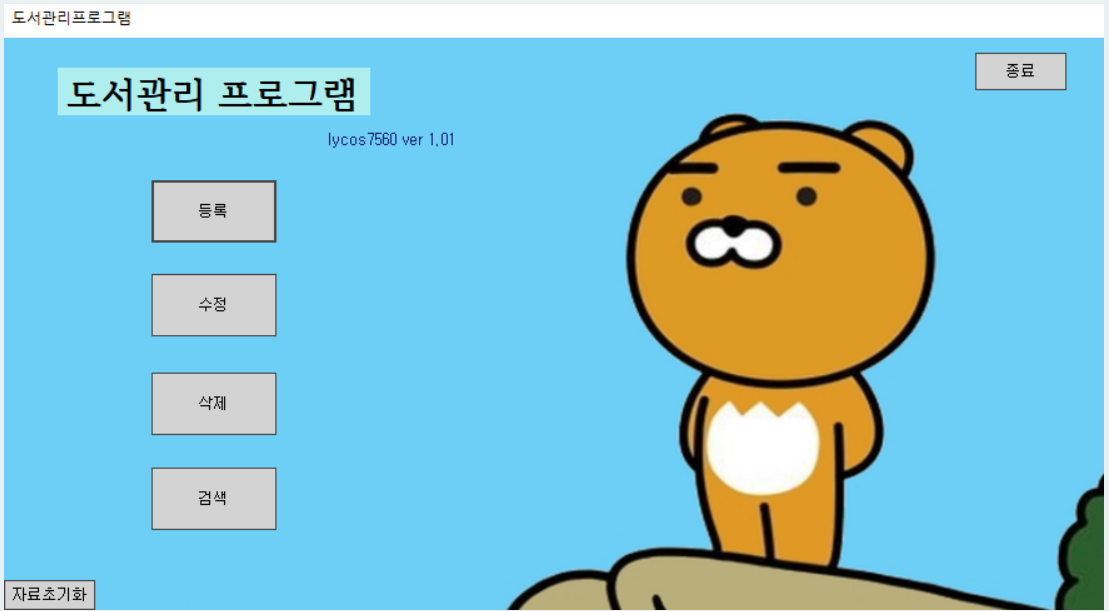
BookData.cs
namespace LibraryManagement { public class BookData { public BookInfo BookInfo; public BookData() { BookInfo = new BookInfo(); } } }
Program.cs
using System; using System.Windows.Forms; namespace LibraryManagement { internal static class Program { /// <summary> /// 해당 애플리케이션의 주 진입점입니다. /// </summary> [STAThread] static void Main() { BookDataManage.instance.BookDataLoing(); Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); Application.Run(new Main()); } } }
Main.cs
using System; using System.Windows.Forms; namespace LibraryManagement { public partial class Main : Form { public Main() { InitializeComponent(); } private void registrationButton_Click(object sender, EventArgs e) { this.Enabled = false; this.Opacity = 0.2; Registration registration = new Registration(this); registration.Show(); } private void button1_Click(object sender, EventArgs e) { this.Enabled = false; this.Opacity = 0.2; Correction correction = new Correction(this); correction.Show(); } private void button2_Click(object sender, EventArgs e) { this.Enabled = false; this.Opacity = 0.2; Remove remove = new Remove(this); remove.Show(); } private void Main_Load(object sender, EventArgs e) { } private void button3_Click(object sender, EventArgs e) { Environment.Exit(0); } private void Serach_Click(object sender, EventArgs e) { this.Enabled = false; this.Opacity = 0.2; Serach serach = new Serach(this); serach.Show(); } private void button4_Click(object sender, EventArgs e) { var check = MessageBox.Show("저장된 자료를 초기화 하시겠습니까?", "확인", MessageBoxButtons.YesNo, MessageBoxIcon.Question); if (check == DialogResult.Yes) { BookDataManage.instance.ReSetData(); } MessageBox.Show("자료가 초기화 되었습니다.", "확인"); } private void label2_Click(object sender, EventArgs e) { } } }
Serach.cs
using System; using System.Collections.Generic; using System.Data; using System.Windows.Forms; namespace LibraryManagement { public partial class Serach : Form { public Main main; public Dictionary<string, BookData> dicBookData = new Dictionary<string, BookData>(); public List<BookData> listSort = new List<BookData>(); DataTable table = new DataTable(); public Serach(Main main) { this.main = main; InitializeComponent(); table.Columns.Add("ISBN", typeof(Int64)); table.Columns.Add("도서명", typeof(string)); table.Columns.Add("출판사", typeof(string)); table.Columns.Add("분류", typeof(string)); table.Columns.Add("가격", typeof(Int32)); table.Columns.Add("등록일", typeof(DateTime)); this.dicBookData = BookDataManage.instance.dicBookData; foreach (KeyValuePair<string, BookData> data in this.dicBookData) { table.Rows.Add($"{Int64.Parse(data.Value.BookInfo.ISBN)}", $"{data.Value.BookInfo.BookName}", $"{data.Value.BookInfo.Publisher}", $"{data.Value.BookInfo.Sort}", $"{data.Value.BookInfo.Price}", $"{data.Value.BookInfo.RegDAta}"); } dataGridView1.DataSource = table; foreach (KeyValuePair<string, BookData> data in this.dicBookData) { this.listSort.Add(data.Value); } this.comboBox2.Enabled = false; this.comboBox3.Enabled = false; this.SerachOK.Enabled = false; } private void OUT_Click(object sender, EventArgs e) { main.Opacity = 1; main.Enabled = true; this.Close(); } private void comboBox2_SelectedIndexChanged(object sender, EventArgs e) { this.comboBox3.Enabled = true; } private void SerachOK_Click(object sender, EventArgs e) { ((DataTable)dataGridView1.DataSource).Rows.Clear(); dataGridView1.Refresh(); string com1 = string.Format(this.comboBox1.SelectedItem.ToString()); string com2 = string.Format(this.comboBox2.SelectedItem.ToString()); string com3 = string.Format(this.comboBox3.SelectedItem.ToString()); if (com1 == "등록날짜") { if (com2 == "버블정렬") { if (com3 == "오름차순") { for (int i = 0; i < this.listSort.Count - 1; i++) { for (int j = i + 1; j < this.listSort.Count; j++) { if (this.listSort[i].BookInfo.RegDAta > this.listSort[j].BookInfo.RegDAta) { BookData temp; temp = listSort[i]; listSort[i] = listSort[j]; listSort[j] = temp; } } } } else if (com3 == "내림차순") { for (int i = 0; i < this.listSort.Count - 1; i++) { for (int j = i + 1; j < this.listSort.Count; j++) { if (this.listSort[i].BookInfo.RegDAta < this.listSort[j].BookInfo.RegDAta) { BookData temp; temp = listSort[i]; listSort[i] = listSort[j]; listSort[j] = temp; } } } } } else if (com2 == "선택정렬") { if (com3 == "오름차순") { for (int i = 0; i < this.listSort.Count; i++) { BookData temp; int index = i; for (int j = i + 1; j < this.listSort.Count; j++) { if (this.listSort[j].BookInfo.RegDAta < this.listSort[index].BookInfo.RegDAta) { index = j; } } if (index == i) { continue; } temp = this.listSort[index]; this.listSort[index] = this.listSort[i]; this.listSort[i] = temp; } } else if (com3 == "내림차순") { for (int i = 0; i < this.listSort.Count; i++) { BookData temp; int index = i; for (int j = i + 1; j < this.listSort.Count; j++) { if (this.listSort[j].BookInfo.RegDAta > this.listSort[index].BookInfo.RegDAta) { index = j; } } if (index == i) { continue; } temp = this.listSort[index]; this.listSort[index] = this.listSort[i]; this.listSort[i] = temp; } } } else if (com2 == "퀵정렬") { if (com3 == "오름차순") { this.listSort = QuickSort1Date(this.listSort, 0, listSort.Count - 1); } else if (com3 == "내림차순") { this.listSort = QuickSort2Date(this.listSort, 0, listSort.Count - 1); } } } else if (com1 == "가격") { if (com2 == "버블정렬") { if (com3 == "오름차순") { for (int i = 0; i < this.listSort.Count - 1; i++) { for (int j = i + 1; j < this.listSort.Count; j++) { if (this.listSort[i].BookInfo.Price > this.listSort[j].BookInfo.Price) { BookData temp; temp = listSort[i]; listSort[i] = listSort[j]; listSort[j] = temp; } } } } else if (com3 == "내림차순") { for (int i = 0; i < this.listSort.Count - 1; i++) { for (int j = i + 1; j < this.listSort.Count; j++) { if (this.listSort[i].BookInfo.Price < this.listSort[j].BookInfo.Price) { BookData temp; temp = listSort[i]; listSort[i] = listSort[j]; listSort[j] = temp; } } } } } else if (com2 == "선택정렬") { if (com3 == "오름차순") { BookData temp; int index; for (int i = 0; i < this.listSort.Count; i++) { index = i; for (int j = i + 1; j < this.listSort.Count; j++) { if (this.listSort[j].BookInfo.Price < this.listSort[index].BookInfo.Price) { index = j; } } if (index == i) { continue; } temp = this.listSort[index]; this.listSort[index] = this.listSort[i]; this.listSort[i] = temp; } } else if (com3 == "내림차순") { BookData temp; int index; for (int i = 0; i < this.listSort.Count; i++) { index = i; for (int j = i + 1; j < this.listSort.Count; j++) { if (this.listSort[j].BookInfo.Price > this.listSort[index].BookInfo.Price) { index = j; } } if (index == i) { continue; } temp = this.listSort[index]; this.listSort[index] = this.listSort[i]; this.listSort[i] = temp; } } } else if (com2 == "퀵정렬") { if (com3 == "오름차순") { this.listSort = QuickSort1Price(this.listSort, 0, listSort.Count - 1); } else if (com3 == "내림차순") { this.listSort = QuickSort2Price(this.listSort, 0, listSort.Count - 1); } } } foreach (BookData data in this.listSort) { table.Rows.Add($"{Int64.Parse(data.BookInfo.ISBN)}", $"{data.BookInfo.BookName}", $"{data.BookInfo.Publisher}", $"{data.BookInfo.Sort}", $"{data.BookInfo.Price}", $"{data.BookInfo.RegDAta}"); } dataGridView1.DataSource = table; } public List<BookData> QuickSort1Date(List<BookData> list, int start, int end) { int startBase = start; int endBase = end; BookData temp; DateTime pivot = list[endBase].BookInfo.RegDAta; while (true) { while (pivot >= list[start].BookInfo.RegDAta && start < end) start++; while (pivot <= list[end].BookInfo.RegDAta && start < end) end--; if (start >= end) break; temp = list[start]; list[start] = list[end]; list[end] = temp; } temp = list[endBase]; list[endBase] = list[start]; list[start] = temp; if (startBase < start) list = QuickSort1Date(list, startBase, start - 1); if (endBase > end) list = QuickSort1Date(list, start + 1, endBase); return list; } public List<BookData> QuickSort2Date(List<BookData> list, int start, int end) { int startBase = start; int endBase = end; BookData temp; DateTime pivot = list[endBase].BookInfo.RegDAta; while (true) { while (pivot <= list[start].BookInfo.RegDAta && start < end) start++; while (pivot >= list[end].BookInfo.RegDAta && start < end) end--; if (start >= end) break; temp = list[start]; list[start] = list[end]; list[end] = temp; } temp = list[endBase]; list[endBase] = list[start]; list[start] = temp; if (startBase < start) list = QuickSort2Date(list, startBase, start - 1); if (endBase > end) list = QuickSort2Date(list, start + 1, endBase); return list; } public List<BookData> QuickSort1Price(List<BookData> list, int start, int end) { int startBase = start; int endBase = end; BookData temp; int pivot = list[endBase].BookInfo.Price; while (true) { while (pivot >= list[start].BookInfo.Price && start < end) start++; while (pivot <= list[end].BookInfo.Price && start < end) end--; if (start >= end) break; temp = list[start]; list[start] = list[end]; list[end] = temp; } temp = list[endBase]; list[endBase] = list[start]; list[start] = temp; if (startBase < start) list = QuickSort1Price(list, startBase, start - 1); if (endBase > end) list = QuickSort1Price(list, start + 1, endBase); return list; } public List<BookData> QuickSort2Price(List<BookData> list, int start, int end) { int startBase = start; int endBase = end; BookData temp; int pivot = list[endBase].BookInfo.Price; while (true) { while (pivot <= list[start].BookInfo.Price && start < end) start++; while (pivot >= list[end].BookInfo.Price && start < end) end--; if (start >= end) break; temp = list[start]; list[start] = list[end]; list[end] = temp; } temp = list[endBase]; list[endBase] = list[start]; list[start] = temp; if (startBase < start) list = QuickSort2Price(list, startBase, start - 1); if (endBase > end) list = QuickSort2Price(list, start + 1, endBase); return list; } private void comboBox1_SelectedIndexChanged(object sender, EventArgs e) { this.comboBox2.Enabled = true; } private void dataGridView1_MouseClick(object sender, MouseEventArgs e) { } private void dataGridView1_CellContentDoubleClick(object sender, DataGridViewCellEventArgs e) { } private void comboBox3_SelectedIndexChanged(object sender, EventArgs e) { this.SerachOK.Enabled = true; } } }
BookDataManage.cs
using System; using System.Collections.Generic; using System.IO; using System.Text; using System.Threading.Tasks; using Newtonsoft.Json; namespace LibraryManagement { public sealed class BookDataManage { public static readonly BookDataManage instance = new BookDataManage(); public Dictionary<string, BookData> dicBookData = new Dictionary<string, BookData>(); private BookDataManage() { } public void BookDataLoing() { if (File.Exists("./book_data.json")) { string json = File.ReadAllText("./book_data.json"); this.dicBookData = JsonConvert.DeserializeObject<Dictionary<string, BookData>>(json); } else { } } public void SaveBookData() { string json = JsonConvert.SerializeObject(this.dicBookData); File.WriteAllText("./book_data.json", json); } public void ReSetData() { this.dicBookData.Clear(); string json = JsonConvert.SerializeObject(this.dicBookData); File.WriteAllText("./book_data.json", json); } } }
BookInfo.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace LibraryManagement { public class BookInfo { public string ISBN { get; set; } public string BookName { get; set; } public string Publisher { get; set; } public string Sort { get; set; } public int Price { get; set; } public DateTime RegDAta { get; set; } } }
Correction.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace LibraryManagement { public partial class Correction : Form { public Main main; public Correction(Main main) { this.main = main; InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { if (BookDataManage.instance.dicBookData.TryGetValue(ISBNTextBook.Text, out BookData data)) { this.BookNameTextBook.Text = data.BookInfo.BookName; this.PublisherTextBook.Text = data.BookInfo.Publisher; this.SeletSortLable.Text = data.BookInfo.Sort; this.PriceTextBook.Text = data.BookInfo.Price.ToString(); this.Search.Enabled = false; this.ISBNTextBook.Enabled = false; this.groupBox1.Enabled = true; } else { MessageBox.Show("해당 값이 없습니다.", "수정 불가"); } } private void OUT_Click(object sender, EventArgs e) { main.Opacity = 1; main.Enabled = true; this.Close(); } private void button2_Click(object sender, EventArgs e) { bool Check = this.ISBNTextBook.Text != "" && this.BookNameTextBook.Text != "" && this.PublisherTextBook.Text != "선택하세요" && this.PriceTextBook.Text != "" && int.TryParse(this.PriceTextBook.Text, out int result); if (Check) { BookData bookData = new BookData(); bookData.BookInfo.ISBN = this.ISBNTextBook.Text; bookData.BookInfo.BookName = this.BookNameTextBook.Text; bookData.BookInfo.Publisher = this.PublisherTextBook.Text; bookData.BookInfo.Sort = this.SeletSortLable.Text; bookData.BookInfo.Price = Convert.ToInt32(this.PriceTextBook.Text); bookData.BookInfo.RegDAta = DateTime.Now; BookDataManage.instance.dicBookData.Remove(bookData.BookInfo.ISBN); BookDataManage.instance.dicBookData.Add(bookData.BookInfo.ISBN, bookData); BookDataManage.instance.SaveBookData(); MessageBox.Show($"수정되었습니다.\n ISBN : {bookData.BookInfo.ISBN} \n 책이름 : {bookData.BookInfo.BookName}\n " + $"출판사 : {bookData.BookInfo.Publisher}\n 분류 : {bookData.BookInfo.Sort}\n 가격 : {bookData.BookInfo.Price} \n 등록일자 : {bookData.BookInfo.RegDAta} ", "확인창"); this.ISBNTextBook.Text = ""; this.BookNameTextBook.Text = ""; this.PublisherTextBook.Text = ""; this.SeletSortLable.Text = "선택하세요"; this.PriceTextBook.Text = ""; this.groupBox1.Enabled = false; this.Search.Enabled = true; this.ISBNTextBook.Enabled = true; } else { MessageBox.Show("빈공간/분류/가격을 확인해주세요", "등록 오류"); } } private void SortComboBox_SelectedIndexChanged(object sender, EventArgs e) { ComboBox cb = (ComboBox)sender; this.SeletSortLable.Text = cb.SelectedItem.ToString(); } } }
Remove.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace LibraryManagement { public partial class Remove : Form { public Main main; public Remove(Main main) { this.main = main; InitializeComponent(); } private void Search_Click(object sender, EventArgs e) { if (BookDataManage.instance.dicBookData.TryGetValue(ISBNTextBook.Text, out BookData data)) { this.BookNameTextBook.Text = data.BookInfo.BookName; this.PublisherTextBook.Text = data.BookInfo.Publisher; this.SeletSortLable.Text = data.BookInfo.Sort; this.PriceTextBook.Text = data.BookInfo.Price.ToString(); this.RemoveButton.Enabled = true; } else { MessageBox.Show("해당 값이 없습니다.", "수정 불가"); } } private void Out_Click(object sender, EventArgs e) { main.Opacity = 1; main.Enabled = true; this.Close(); } private void RemoveButton_Click(object sender, EventArgs e) { const string message = "정말 삭제하시겠습니까?"; const string caption = "확인 메시지"; var check = MessageBox.Show(message, caption,MessageBoxButtons.YesNo,MessageBoxIcon.Question); if (check == DialogResult.Yes) { bool Check = this.ISBNTextBook.Text != "" && this.BookNameTextBook.Text != "" && this.PublisherTextBook.Text != "선택하세요" && this.PriceTextBook.Text != "" && int.TryParse(this.PriceTextBook.Text, out int result); if (Check) { BookData bookData = new BookData(); bookData.BookInfo.ISBN = this.ISBNTextBook.Text; bookData.BookInfo.BookName = this.BookNameTextBook.Text; bookData.BookInfo.Publisher = this.PublisherTextBook.Text; bookData.BookInfo.Sort = this.SeletSortLable.Text; bookData.BookInfo.Price = Convert.ToInt32(this.PriceTextBook.Text); bookData.BookInfo.RegDAta = DateTime.Now; BookDataManage.instance.dicBookData.Remove(bookData.BookInfo.ISBN); BookDataManage.instance.SaveBookData(); this.ISBNTextBook.Text = ""; this.BookNameTextBook.Text = ""; this.PublisherTextBook.Text = ""; this.SeletSortLable.Text = "선택하세요"; this.PriceTextBook.Text = ""; this.RemoveButton.Enabled = false; } else { MessageBox.Show("해당 책을 찾을 수 없습니다.", "오류"); } } } } }