Dependency Inversion Principle(DIP, 의존성 역전 원리)
The direction of dependency within the application should be in the direction of abstraction, not implementation details.
애플리케이션 내의 종속성 방향은 구현 세부 사항이 아닌 추상화 방향이어야 합니다.
Most applications are written such that compile-time dependency flows in the direction of runtime execution, producing a direct dependency graph.
대부분의 애플리케이션은 컴파일 타임 종속성이 런타임 실행 방향으로 흐르도록 작성되어 직접적인 종속성 그래프를 생성합니다.
That is, if class A calls a method of class B and class B calls a method of class C, then at compile time class A will depend on class B, and class B will depend on class C.
즉, class A가 class B의 메서드를 호출하고 class B가 class C의 메서드를 호출하는 경우 컴파일 타임에 class A는 class B에 종속되고 class B는 class C에 종속됩니다.
코드 구조에서 세부 구현 내용에 의존하지 말고, 더 추상적이고 일반적인 개념에 의존해야 한다는 뜻
이렇게 하면 코드가 더 유연해지고, 변경하기 쉽고, 재사용하기도 쉬워진다.
예를 들어, 어떤 기능을 직접 호출하는 것보다 그 기능을 일반적으로 설명하는 인터페이스를 사용하는 것이 더 좋다는 의미)
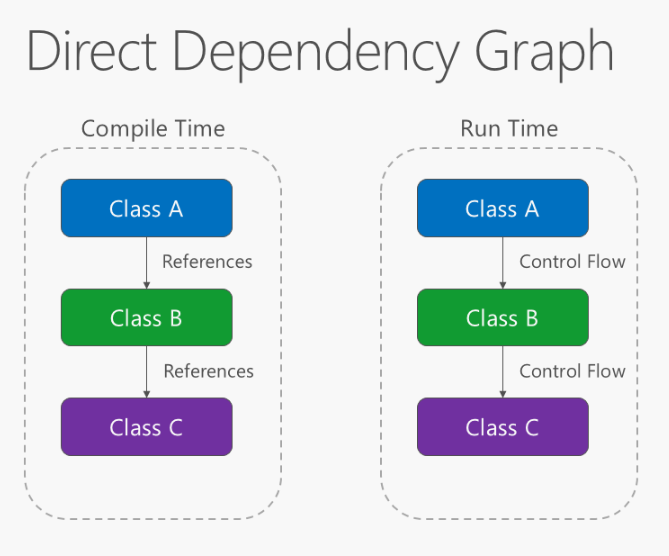
Applying the dependency inversion principle allows A to call methods on an abstraction that B implements, making it possible for A to call B at run time, but for B to depend on an interface controlled by A at compile time (thus, inverting the typical compile-time dependency).
종속성 반전 원칙을 적용하면 A가 B가 구현하는 추상화에 대한 메서드를 호출할 수 있으므로 A가 런타임에 B를 호출할 수 있지만 B는 컴파일 타임에 A에 의해 제어되는 인터페이스에 의존할 수 있습니다
(따라서 일반적인 컴파일 타임 종속성을 반전시킵니다).
At run time, the flow of program execution remains unchanged, but the introduction of interfaces means that different implementations of these interfaces can easily be plugged in.
런타임에 프로그램 실행의 흐름은 변경되지 않지만 인터페이스의 도입은 이러한 인터페이스의 다른 구현을 쉽게 연결할 수 있음을 의미합니다.
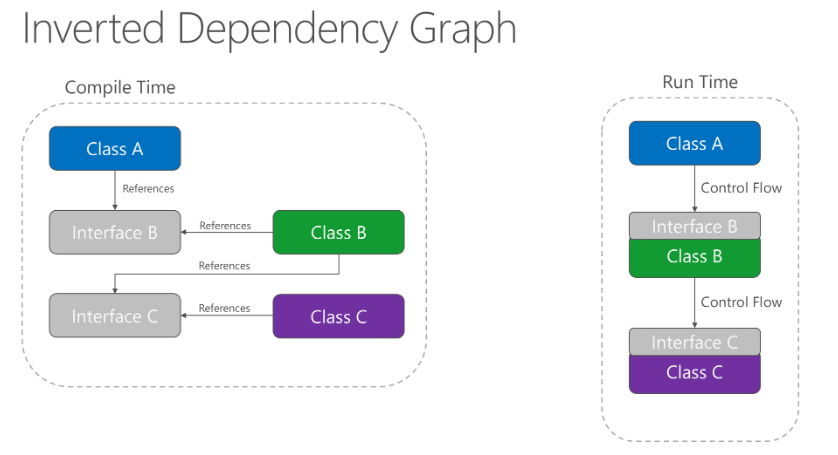
Dependency inversion is a key part of building loosely coupled applications, since implementation details can be written to depend on and implement higher-level abstractions, rather than the other way around.
종속성 반전은 느슨하게 결합된 애플리케이션을 구축하는 데 있어 중요한 부분인데, 그 이유는 구현 세부 정보를 작성하여 더 높은 수준의 추상화에 의존하고 구현하도록 할 수 있기 때문입니다.
The resulting applications are more testable, modular, and maintainable as a result.
그 결과 응용 프로그램은 더 쉽게 테스트할 수 있고, 모듈화되며, 유지 관리가 더 용이합니다.
The practice of dependency injection is made possible by following the dependency inversion principle.
종속성 주입의 연습은 종속성 반전 원칙을 따름으로써 가능합니다.
애플리케이션의 의존성을 구체적인 구현이 아니라 추상화된 개념에 맞추는 것
대부분의 애플리케이션은 A 클래스가 B 클래스를, B 클래스가 C 클래스를 호출하는 형태로 작성됩니다.
이는 A가 B에, B가 C에 의존하게 만든다는 뜻입니다. 종속성 반전 원칙을 적용하면 A는 B의 구체적인 구현이 아니라 B가 구현하는 추상 개념에 의존하게 됩니다.
이렇게 하면 코드를 더 쉽게 테스트할 수 있고, 변경 및 유지보수가 용이해집니다.
간단히 말해, 코드를 유연하고 재사용 가능하게 만들어줍니다.
종속성 반전 적용 전
// 엔진 클래스 public class Engine { public void Start() { Console.WriteLine("Engine starts."); } } // 자동차 클래스 public class Car { private Engine _engine = new Engine(); public void Start() { _engine.Start(); Console.WriteLine("Car starts."); } } // 메인 프로그램 public class Program { public static void Main(string[] args) { Car car = new Car(); car.Start(); } }
종속성 반전 적용 후
// 인터페이스 정의 public interface IEngine { void Start(); } // 엔진 클래스 public class Engine : IEngine { public void Start() { Console.WriteLine("Engine starts."); } } // 전기 엔진 클래스 public class ElectricEngine : IEngine { public void Start() { Console.WriteLine("Electric engine starts."); } } // 자동차 클래스 public class Car { private IEngine _engine; public Car(IEngine engine) { _engine = engine; } public void Start() { _engine.Start(); Console.WriteLine("Car starts."); } } // 메인 프로그램 public class Program { public static void Main(string[] args) { IEngine engine = new Engine(); Car car = new Car(engine); car.Start(); IEngine electricEngine = new ElectricEngine(); Car electricCar = new Car(electricEngine); electricCar.Start(); } }
İstanbul da kaçak su tespiti Pratik Çözüm: “Tesisat sorunlarına pratik çözümler sundular. https://www.buzzbii.com/ustaelektrikci