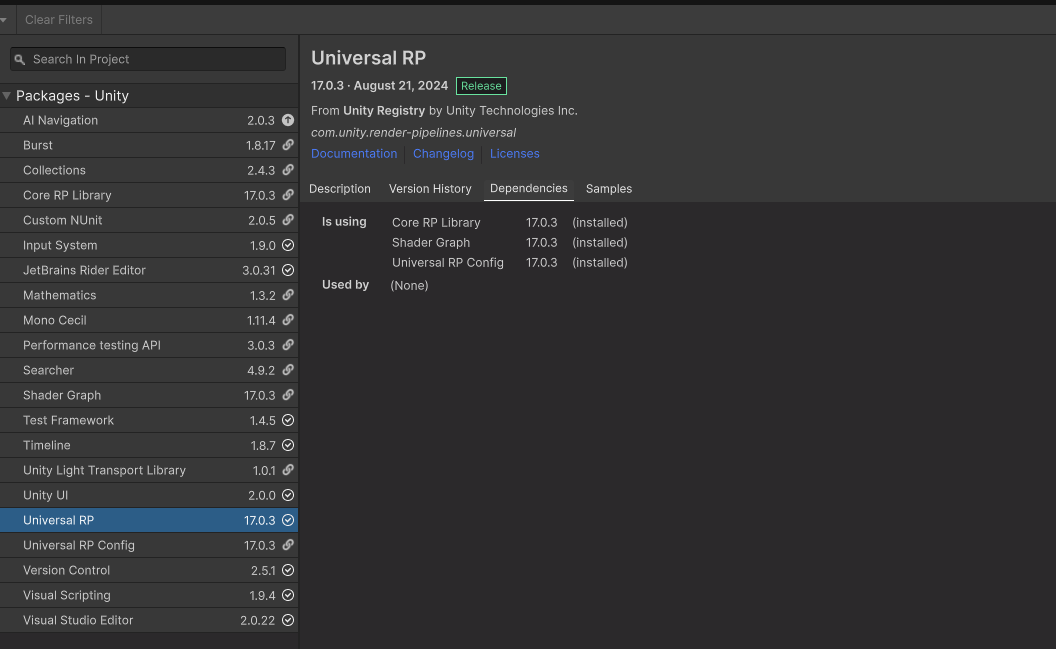
Universal RP 17.0.3
include “Packages/com.unity.render-pipelines.universal/ShaderLibrary/Core.hlsl” 내부에 input이 포함됨
include “Packages/com.unity.render-pipelines.universal/ShaderLibrary/Input.hlsl”
Input.hlsl
#ifndef UNIVERSAL_INPUT_INCLUDED #define UNIVERSAL_INPUT_INCLUDED // URP package specific shader input variables and defines. // Unity Engine specific built-in shader input variables are defined in .universal/ShaderLibrary/UnityInput.hlsl #include "Packages/com.unity.render-pipelines.universal-config/Runtime/ShaderConfig.cs.hlsl" #define MAX_VISIBLE_LIGHTS_UBO 32 #define MAX_VISIBLE_LIGHTS_SSBO 256 // Keep in sync with RenderingUtils.useStructuredBuffer #define USE_STRUCTURED_BUFFER_FOR_LIGHT_DATA 0 #include "Packages/com.unity.render-pipelines.universal/ShaderLibrary/ShaderTypes.cs.hlsl" #include "Packages/com.unity.render-pipelines.universal/ShaderLibrary/Deprecated.hlsl" // Must match: UniversalRenderPipeline.maxVisibleAdditionalLights #if defined(SHADER_API_MOBILE) && defined(SHADER_API_GLES30) #define MAX_VISIBLE_LIGHTS MAX_VISIBLE_LIGHT_COUNT_LOW_END_MOBILE // WebGPU's minimal limits are based on mobile rather than desktop, so it will need to assume mobile. #elif defined(SHADER_API_MOBILE) || (defined(SHADER_API_GLCORE) && !defined(SHADER_API_SWITCH)) || defined(SHADER_API_GLES3) || defined(SHADER_API_WEBGPU) // Workaround because SHADER_API_GLCORE is also defined when SHADER_API_SWITCH is #define MAX_VISIBLE_LIGHTS MAX_VISIBLE_LIGHT_COUNT_MOBILE #else #define MAX_VISIBLE_LIGHTS MAX_VISIBLE_LIGHT_COUNT_DESKTOP #endif // Match with values in UniversalRenderPipeline.cs #define MAX_ZBIN_VEC4S 1024 #if MAX_VISIBLE_LIGHTS <= 16 #define MAX_LIGHTS_PER_TILE 32 #define MAX_TILE_VEC4S 1024 #define MAX_REFLECTION_PROBES 16 #elif MAX_VISIBLE_LIGHTS <= 32 #define MAX_LIGHTS_PER_TILE 32 #define MAX_TILE_VEC4S 1024 #define MAX_REFLECTION_PROBES 32 #else #define MAX_LIGHTS_PER_TILE MAX_VISIBLE_LIGHTS #define MAX_TILE_VEC4S 4096 #define MAX_REFLECTION_PROBES 64 #endif struct InputData { float3 positionWS; float4 positionCS; float3 normalWS; half3 viewDirectionWS; float4 shadowCoord; half fogCoord; half3 vertexLighting; half3 bakedGI; float2 normalizedScreenSpaceUV; half4 shadowMask; half3x3 tangentToWorld; #if defined(DEBUG_DISPLAY) half2 dynamicLightmapUV; half2 staticLightmapUV; float3 vertexSH; half3 brdfDiffuse; half3 brdfSpecular; // Mipmap Streaming Debug float2 uv; uint mipCount; // texelSize : // x = 1 / width // y = 1 / height // z = width // w = height float4 texelSize; // mipInfo : // x = quality settings minStreamingMipLevel // y = original mip count for texture // z = desired on screen mip level // w = loaded mip level float4 mipInfo; // streamInfo : // x = streaming priority // y = time stamp of the latest texture upload // z = streaming status // w = 0 float4 streamInfo; float3 originalColor; #endif }; /////////////////////////////////////////////////////////////////////////////// // Constant Buffers // /////////////////////////////////////////////////////////////////////////////// half4 _GlossyEnvironmentColor; half4 _SubtractiveShadowColor; half4 _GlossyEnvironmentCubeMap_HDR; TEXTURECUBE(_GlossyEnvironmentCubeMap); SAMPLER(sampler_GlossyEnvironmentCubeMap); #define _InvCameraViewProj unity_MatrixInvVP float4 _ScaledScreenParams; // x = Mip Bias // y = 2.0 ^ [Mip Bias] float2 _GlobalMipBias; // 1.0 if it's possible for AlphaToMask to be enabled for this draw and 0.0 otherwise float _AlphaToMaskAvailable; float4 _MainLightPosition; // In Forward+, .a stores whether the main light is using subtractive mixed mode. half4 _MainLightColor; half4 _MainLightOcclusionProbes; uint _MainLightLayerMask; // x: SSAO Enabled/Disabled (Needed for situations when OFF keyword is stripped out but feature disabled in runtime) // yz are currently unused // w: directLightStrength half4 _AmbientOcclusionParam; half4 _AdditionalLightsCount; uint _RenderingLayerMaxInt; float _RenderingLayerRcpMaxInt; // Screen coord override. float4 _ScreenCoordScaleBias; float4 _ScreenSizeOverride; uint _EnableProbeVolumes; #if USE_FORWARD_PLUS float4 _FPParams0; float4 _FPParams1; float4 _FPParams2; #define URP_FP_ZBIN_SCALE (_FPParams0.x) #define URP_FP_ZBIN_OFFSET (_FPParams0.y) #define URP_FP_PROBES_BEGIN ((uint)_FPParams0.z) // Directional lights would be in all clusters, so they don't go into the cluster structure. // Instead, they are stored first in the light buffer. #define URP_FP_DIRECTIONAL_LIGHTS_COUNT ((uint)_FPParams0.w) // Scale from screen-space UV [0, 1] to tile coordinates [0, tile resolution]. #define URP_FP_TILE_SCALE ((float2)_FPParams1.xy) #define URP_FP_TILE_COUNT_X ((uint)_FPParams1.z) #define URP_FP_WORDS_PER_TILE ((uint)_FPParams1.w) #define URP_FP_ZBIN_COUNT ((uint)_FPParams2.x) #define URP_FP_TILE_COUNT ((uint)_FPParams2.y) #endif #if USE_STRUCTURED_BUFFER_FOR_LIGHT_DATA StructuredBuffer<LightData> _AdditionalLightsBuffer; StructuredBuffer<int> _AdditionalLightsIndices; #else // GLES3 causes a performance regression in some devices when using CBUFFER. #ifndef SHADER_API_GLES3 CBUFFER_START(AdditionalLights) #endif float4 _AdditionalLightsPosition[MAX_VISIBLE_LIGHTS]; // In Forward+, .a stores whether the light is using subtractive mixed mode. half4 _AdditionalLightsColor[MAX_VISIBLE_LIGHTS]; half4 _AdditionalLightsAttenuation[MAX_VISIBLE_LIGHTS]; half4 _AdditionalLightsSpotDir[MAX_VISIBLE_LIGHTS]; half4 _AdditionalLightsOcclusionProbes[MAX_VISIBLE_LIGHTS]; float _AdditionalLightsLayerMasks[MAX_VISIBLE_LIGHTS]; // we want uint[] but Unity api does not support it. #ifndef SHADER_API_GLES3 CBUFFER_END #endif #endif #if USE_FORWARD_PLUS CBUFFER_START(urp_ZBinBuffer) float4 urp_ZBins[MAX_ZBIN_VEC4S]; CBUFFER_END CBUFFER_START(urp_TileBuffer) float4 urp_Tiles[MAX_TILE_VEC4S]; CBUFFER_END TEXTURE2D(urp_ReflProbes_Atlas); float urp_ReflProbes_Count; // 2023.3 Deprecated. This is for backwards compatibility. Remove in the future. #define samplerurp_ReflProbes_Atlas sampler_LinearClamp #ifndef SHADER_API_GLES3 CBUFFER_START(urp_ReflectionProbeBuffer) #endif float4 urp_ReflProbes_BoxMax[MAX_REFLECTION_PROBES]; // w contains the blend distance float4 urp_ReflProbes_BoxMin[MAX_REFLECTION_PROBES]; // w contains the importance float4 urp_ReflProbes_ProbePosition[MAX_REFLECTION_PROBES]; // w is positive for box projection, |w| is max mip level float4 urp_ReflProbes_MipScaleOffset[MAX_REFLECTION_PROBES * 7]; #ifndef SHADER_API_GLES3 CBUFFER_END #endif #endif #define UNITY_MATRIX_M unity_ObjectToWorld #define UNITY_MATRIX_I_M unity_WorldToObject #define UNITY_MATRIX_V unity_MatrixV #define UNITY_MATRIX_I_V unity_MatrixInvV #define UNITY_MATRIX_P OptimizeProjectionMatrix(glstate_matrix_projection) #define UNITY_MATRIX_I_P unity_MatrixInvP #define UNITY_MATRIX_VP unity_MatrixVP #define UNITY_MATRIX_I_VP unity_MatrixInvVP #define UNITY_MATRIX_MV mul(UNITY_MATRIX_V, UNITY_MATRIX_M) #define UNITY_MATRIX_T_MV transpose(UNITY_MATRIX_MV) #define UNITY_MATRIX_IT_MV transpose(mul(UNITY_MATRIX_I_M, UNITY_MATRIX_I_V)) #define UNITY_MATRIX_MVP mul(UNITY_MATRIX_VP, UNITY_MATRIX_M) #define UNITY_PREV_MATRIX_M unity_MatrixPreviousM #define UNITY_PREV_MATRIX_I_M unity_MatrixPreviousMI // Note: #include order is important here. // UnityInput.hlsl must be included before UnityInstancing.hlsl, so constant buffer // declarations don't fail because of instancing macros. // UniversalDOTSInstancing.hlsl must be included after UnityInstancing.hlsl #include "Packages/com.unity.render-pipelines.universal/ShaderLibrary/UnityInput.hlsl" #include "Packages/com.unity.render-pipelines.core/ShaderLibrary/UnityInstancing.hlsl" #include "Packages/com.unity.render-pipelines.universal/ShaderLibrary/UniversalDOTSInstancing.hlsl" // VFX may also redefine UNITY_MATRIX_M / UNITY_MATRIX_I_M as static per-particle global matrices. #ifdef HAVE_VFX_MODIFICATION #include "Packages/com.unity.visualeffectgraph/Shaders/VFXMatricesOverride.hlsl" #endif #include "Packages/com.unity.render-pipelines.core/ShaderLibrary/SpaceTransforms.hlsl" #endif
1. 헤더 정의 및 보호구문
#ifndef UNIVERSAL_INPUT_INCLUDED #define UNIVERSAL_INPUT_INCLUDED
헤더 파일을 여러 번 포함하는 것을 방지
UNIVERSAL_INPUT_INCLUDED
가 정의되지 않은 경우에만 코드가 실행되도록 합니다.
2. 패키지 및 설정 파일 포함
#include "Packages/com.unity.render-pipelines.universal-config/Runtime/ShaderConfig.cs.hlsl"
URP의 설정 및 구성을 정의하는 파일을 포함
이 파일에는 URP에서 사용하는 여러 가지 상수 값과 설정이 정의되어 있습니다.
3. 최대 조명 및 타일링 설정
#define MAX_VISIBLE_LIGHTS_UBO 32 #define MAX_VISIBLE_LIGHTS_SSBO 256
UBO(Uniform Buffer Object)와 SSBO(Shader Storage Buffer Object)에 사용할 수 있는 최대 조명 개수를 정의
MAX_VISIBLE_LIGHTS_UBO: UBO 방식으로 사용할 수 있는 최대 조명 수 (32).
MAX_VISIBLE_LIGHTS_SSBO: SSBO 방식으로 사용할 수 있는 최대 조명 수 (256).
4. 조명 데이터 저장 방법 결정
#define USE_STRUCTURED_BUFFER_FOR_LIGHT_DATA 0
0으로 설정되어 있으며, 이는 Structured Buffer가 아닌 Constant Buffer를 사용하여 조명 데이터를 저장함을 의미합니다.
5. 플랫폼에 따른 최대 조명 설정
// Must match: UniversalRenderPipeline.maxVisibleAdditionalLights #if defined(SHADER_API_MOBILE) && defined(SHADER_API_GLES30) #define MAX_VISIBLE_LIGHTS MAX_VISIBLE_LIGHT_COUNT_LOW_END_MOBILE // WebGPU's minimal limits are based on mobile rather than desktop, so it will need to assume mobile. #elif defined(SHADER_API_MOBILE) || (defined(SHADER_API_GLCORE) && !defined(SHADER_API_SWITCH)) || defined(SHADER_API_GLES3) || defined(SHADER_API_WEBGPU) // Workaround because SHADER_API_GLCORE is also defined when SHADER_API_SWITCH is #define MAX_VISIBLE_LIGHTS MAX_VISIBLE_LIGHT_COUNT_MOBILE #else #define MAX_VISIBLE_LIGHTS MAX_VISIBLE_LIGHT_COUNT_DESKTOP #endif
플랫폼에 따라 최대 조명 수를 다르게 설정합니다.
모바일 플랫폼에서는 자원을 덜 사용하도록 제한을 두며, 데스크탑에서는 더 많은 조명을 허용합니다.
6. 입력 데이터 구조체 (InputData)
struct InputData { float3 positionWS; float4 positionCS; float3 normalWS; half3 viewDirectionWS; float4 shadowCoord; half fogCoord; half3 vertexLighting; half3 bakedGI; float2 normalizedScreenSpaceUV; half4 shadowMask; half3x3 tangentToWorld; };
InputData 구조체는 Shader에서 필요한 여러 데이터를 모아둔 것입니다.
positionWS
: 월드 좌표계에서의 정점 위치.positionCS
: 클립 좌표계에서의 정점 위치.normalWS
: 월드 좌표계에서의 법선 벡터.viewDirectionWS
: 월드 좌표계에서의 시선 방향.shadowCoord
: 그림자 좌표.vertexLighting
: 정점 기반의 조명.bakedGI
: 프리컴퓨티드 전역 조명.normalizedScreenSpaceUV
: 화면 공간 UV 좌표.shadowMask
: 그림자 마스크.tangentToWorld
: 탄젠트 공간에서 월드 공간으로 변환하는 행렬.
7. 그 외 입력 구조체 (DEBUG_DISPLAY 포함)
DEBUG_DISPLAY가 정의되어 있는 경우 추가적인 디버그용 데이터를 받습니다.
- 동적, 정적 라이트맵 UV 좌표, 텍스처 스트리밍 관련 정보 등을 포함합니다.
8. 상수 버퍼 및 조명 데이터
half4 _GlossyEnvironmentColor; half4 _SubtractiveShadowColor; half4 _GlossyEnvironmentCubeMap_HDR; TEXTURECUBE(_GlossyEnvironmentCubeMap); SAMPLER(sampler_GlossyEnvironmentCubeMap);
환경 반사, 그림자 색상, 큐브맵 반사 등과 관련된 상수 값들이 정의됩니다.
텍스처와 샘플러도 정의됩니다.
9. 주 조명 및 추가 조명 데이터
float4 _MainLightPosition; half4 _MainLightColor; float4 _AdditionalLightsPosition[MAX_VISIBLE_LIGHTS]; half4 _AdditionalLightsColor[MAX_VISIBLE_LIGHTS];
주 조명과 추가 조명에 대한 데이터를 저장합니다.
각 조명에 대해 위치, 색상, 감쇠(Attenuation), 스포트라이트 방향 등이 포함됩니다.
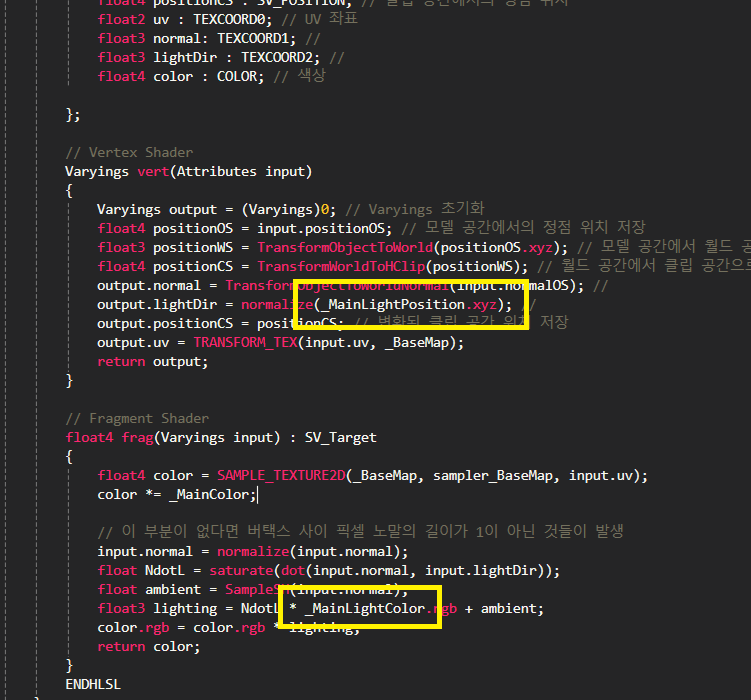
10. 타일 기반 및 반사 프로브 처리 (Forward Plus)
CBUFFER_START(urp_TileBuffer) float4 urp_Tiles[MAX_TILE_VEC4S]; CBUFFER_END
타일 기반 조명 처리 및 반사 프로브에 대한 데이터를 정의합니다.
이는 Forward Plus 렌더링에서 사용됩니다.타일당 조명 수, 타일 크기, 반사 프로브의 위치 등을 정의합니다.
11. 매트릭스 정의
#define UNITY_MATRIX_M unity_ObjectToWorld #define UNITY_MATRIX_I_M unity_WorldToObject
객체에서 월드로 변환하는 매트릭스와 반대로 월드에서 객체로 변환하는 매트릭스 등 다양한 매트릭스가 정의됩니다.
12. 기타 패키지 포함
#include "Packages/com.unity.render-pipelines.universal/ShaderLibrary/UnityInput.hlsl" #include "Packages/com.unity.render-pipelines.core/ShaderLibrary/UnityInstancing.hlsl"
Unity의 인스턴싱 및 DOTS 관련 쉐이더 라이브러리를 포함하여 인스턴싱 처리 및 VFX 처리 관련 기능을 지원합니다.
이 코드는 Universal Render Pipeline에서 사용하는 다양한 조명, 타일 기반 조명 처리, 반사 처리 및 그림자 처리를 위한 입력 데이터를 처리하는 방법을 정의합니다.