HLSL in Unity
https://docs.unity3d.com/2023.2/Documentation/Manual/SL-ShaderPrograms.html
- HLSL in Unity
- Preprocessor directives in HLSL (HLSL의 전처리기 지시어)
- include and include_with_pragmas directives in HLSL(HLSL의 include 및 include_with_pragmas 지시어)
- Provide information to the shader compiler in HLSL(HLSL의 shader compiler에 정보 제공)
- Targeting shader models and GPU features in HLSL(HLSL의 셰이더 모델 및 GPU 기능 타겟팅)
- Targeting graphics APIs and platforms in HLSL(HLSL에서 그래픽 API 및 플랫폼 타겟팅)
- Declaring and using shader keywords in HLSL(HLSL에서 쉐이더 키워드 선언 및 사용)
- Shader semantics (쉐이더 의미론)
- Accessing shader properties in Cg/HLSL(Cg/HLSL에서 셰이더 속성 접근)
- Providing vertex data to vertex programs (버텍스 프로그램에 버텍스 데이터 제공)
- Built-in shader include files
In Unity, you use the HLSL programming language to write shader programs.
(Unity에서는 HLSL 프로그래밍 언어를 사용하여 셰이더를 작성합니다.)
This section of the manual includes information on using HLSL in a Unity-specific way.
(이 메뉴얼의 섹션은 HLSL을 Unity-specific 방식으로 사용하는 방법이 포함되어있습니다.
* Unity-specific => Unity 엔진에서 HLSL을 사용하는 특수한 규칙이나 패턴을 의미,
Unity는 기본적인 HLSL 문법과 개념을 사용하지만, Shader를 작성할 때 ShaderLab 시스템과 통합하여 사용하는 방식을 채택하고 있음.
렌더링 파이프라인과 호환되도록 설계된 특정 구조와 방법)
For general information on writing HLSL, see Microsoft’s HLSL documentation.
(HLSL 작성에 대한 일반적인 정보는 Microsoft의 HLSL 문서를 참조하세요.)
Note: Unity originally used the Cg language, hence the name of some of Unity’s keywords (CGPROGRAM) and file extensions (.cginc).
(참고: Unity는 원래 Cg 언어를 사용했기 때문에 Unity의 일부 키워드(CGPROGRAM)와 파일 확장자(.cginc)의 이름을 사용했습니다.)
Unity no longer uses Cg, but these names are still in use.
(유니티는 더 이상 Cg를 사용하지 않지만 이러한 이름은 여전히 사용되고 있습니다.)
Adding HLSL code to your ShaderLab code
(ShaderLab code에 HLSL 코드 추가)
You place your HLSL code inside code blocks in your ShaderLab code.
ShaderLab code 블록 안에 HLSL code를 넣습니다.
They usually look like this:
보통 아래와 같이 보입니다.
Pass { // ... the usual pass state setup ... HLSLPROGRAM // compilation directives for this snippet, e.g.: #pragma vertex vert #pragma fragment frag // the shader program itself ENDHLSL // ... the rest of pass ... }
For more information on shader code blocks, see ShaderLab: adding shader programs.
(shader code blocks에 대한 자세한 내용은 ShaderLab: shader 프로그램 추가를 참조하세요.)
HLSL syntax (HLSL 구문)
HLSL has two syntaxes: a legacy DirectX 9-style syntax, and a more modern DirectX 10+ style syntax.
(HLSL에는 기존 DirectX 9 스타일 syntax와 최신 DirectX 10+ 스타일 syntax의 두 가지 syntax가 있습니다.)
The difference is mostly in how texture sampling functions work:
(차이점은 대부분 텍스처 샘플링 기능의 작동 방식에 있습니다:)
- – The legacy syntax uses sampler2D, tex2D() and similar functions. This syntax works on all platforms.
(기존 구문은 sampler2D, tex2D() 및 유사한 기능을 사용합니다. 이 syntax는 모든 플랫폼에서 작동합니다.) - – The DX10+ syntax uses Texture2D, SamplerState and .Sample() functions.
(DX10+ 구문은 Texture2D, SamplerState 및 .Sample() 함수를 사용합니다.)
Some forms of this syntax do not work on OpenGL platforms, because textures and samplers are not different objects in OpenGL.
(textures와 samplers 는 OpenGL에서 서로 다른 개체가 아니기 때문에 이 syntax의 일부 형태는 OpenGL 플랫폼에서 작동하지 않습니다.)
Unity provides shader libraries that contain preprocessor macros to help you manage these differences.
(Unity는 이러한 차이점을 관리하는 데 도움이 되는 전처리 매크로(preprocessor macros)가 포함된 shader libraries를 제공합니다.)
For more information, see Built-in shader macros.
자세한 내용은 Built-in shader macros를 참조하세요.
Preprocessor directives in HLSL
(HLSL의 전처리기 지시어)
https://docs.unity3d.com/2023.2/Documentation/Manual/shader-preprocessor-directives.html
Internally, shader compilation has multiple stages.
(내부적으로 shader 컴파일에는 여러 단계가 있습니다.)
The first stage is preprocessing, where a program called the preprocessor prepares the code for compilation.
(첫 번째 단계는 전처리 단계로, 전처리라는 프로그램이 코드를 컴파일을 위해 준비합니다.)
Preprocessor directives are instructions for the preprocessor.
(전처리기 지시어는 전처기기에 대한 지시 사항입니다.)
This section of the manual contains information on Unity-specific ways of working with HLSL preprocessor directives, and HLSL preprocessor directives that are unique to Unity.
(이 manual의 이 section에는 Unity-specific 방식으로 HLSL 전처리기 지시어를 사용하는 방법과 Unity에 고유한 HLSL 전처리기 지시어에 대한 정보가 포함되어 있습니다.)
It does not contain exhaustive documentation on all the preprocessor directives that HLSL supports, or general information on working with preprocessor directives in HLSL.
(HLSL이 지원하는 모든 전처리기 지시어에 대한 포괄적인 문서나 HLSL의 전처리기 지시어를 사용하는 방법에 대한 일반적인 정보는 포함되어 있지 않습니다.)
For that information, see the HLSL documentation: Preprocessor directives (HLSL)
(해당 정보는 HLSL 문서의 전처리기 지시어(Preprocessor directives) 섹션을 참조하세요. : Preprocessor directives (HLSL))
Page | Description |
---|---|
include and include_with_pragmas directives in HLSL (HLSL에서 include 및 include_with_pragmas 지시어) | Working with #include and the Unity-specific #include_with_pragmas directives in HLSL in Unity.(Unity에서 HLSL에서 #include 와 Unity-specific #include_with_pragmas 지시어를 사용하는 방법에 대한 설명.) |
Provide information to the shader compiler in HLSL (HLSL에서 셰이더 컴파일러에 정보 제공하기) | Working with #pragma directives and the #define_for_platform_compiler directive in HLSL in Unity.(Unity에서 HLSL에서 #pragma 지시어와 #define_for_platform_compiler 지시어를 사용하는 방법에 대한 설명.) |
Targeting shader models and GPU features in HLSL (HLSL에서 셰이더 모델 및 GPU 기능 타겟팅하기) | sing #pragma directives to indicate that your shader requires certain GPU features.(셰이더가 특정 GPU 기능을 요구함을 나타내기 위해 #pragma 지시어를 사용하는 방법에 대한 설명.) |
Targeting graphics APIs and platforms in HLSL (HLSL에서 그래픽스 API 및 플랫폼 타겟팅하기) | Using #pragma directives to target specific graphics API and platforms.(특정 그래픽스 API와 플랫폼을 타겟팅하기 위해 #pragma 지시어를 사용하는 방법에 대한 설명.) |
Declaring and using shader keywords in HLSL HLSL에서 셰이더 키워드 선언 및 사용하기 | Using #pragma directives to declare shader keywords and #if directives to indicate that code depends on the state of shader keywords.(셰이더 키워드를 선언하기 위해 #pragma 지시어를 사용하고, 셰이더 키워드의 상태에 따라 코드가 의존하는 것을 나타내기 위해 #if 지시어를 사용하는 방법에 대한 설명.) |
include and include_with_pragmas directives in HLSL
(HLSL의 include
및 include_with_pragmas
지시어)
https://docs.unity3d.com/2023.2/Documentation/Manual/shader-include-directives.html
In HLSL, #include
directives are a type of preprocessor directive.
(HLSL에서 #include
지시어는 전처리기 지시어의 일종입니다.)
They instruct the compiler to include the contents of one HLSL file inside another.
(이들은 컴파일러에게 하나의 HLSL 파일의 내용을 다른 파일에 포함하도록 지시합니다.)
The file that they include is called an include file.
(이때 포함되는 파일을 include file이라고 합니다.)
In Unity, regular #include
directives work the same as in standard HLSL.
(Unity에서 일반적인 #include
지시어는 표준 HLSL과 동일하게 작동합니다.)
For more information on regular #include
directives, see the HLSL documentation: include Directive.
일반적인 #include
지시어에 대한 자세한 정보는 HLSL 문서의 include Directive
를 참조하세요.
Unity also provides an additional, Unity-specific #include_with_pragmas
directive.
(Unity는 추가적으로 Unity-specific #include_with_pragmas
지시어를 제공합니다.)
The #include_with_pragmas
directive works the same as a regular #include
directive, but it also allows you to use #pragma
directives in the include file.
(#include_with_pragmas
지시어는 일반 #include
지시어와 동일하게 작동하지만, 포함 파일 내에서 #pragma
지시어를 사용할 수 있게 해줍니다.)
This means that the #include_with_pragmas
directive allows you to share #pragma
directives between multiple files.
(즉, #include_with_pragmas
지시어를 사용하면 여러 파일 간에 #pragma
지시어를 공유할 수 있습니다.)
Using the include_with_pragmas directive
(include_with_pragmas
지시어 사용하기)
Note: To use #include_with_pragmas
directives, you must enable the Caching Shader Preprocessor.
(참고: #include_with_pragmas
지시어를 사용하려면 Caching Shader Preprocessor를 활성화해야 합니다.)
This example demonstrates how to use the Unity-specific #include_with_pragmas
directive to enable a common workflow improvement: the ability to toggle shader debugging on and off for multiple shaders, without needing to edit every shader source file every time.
(다음 예시는 Unity-specific #include_with_pragmas
지시어를 사용하여 셰이더 디버깅을 켜고 끌 수 있는 일반적인 작업 흐름 개선 방법을 보여줍니다.
이를 통해 여러 셰이더의 디버깅을 설정할 때마다 매번 셰이더 소스 파일을 수정할 필요가 없습니다.)
The following line demonstrates the contents of the include file.
(다음 줄은 포함 파일의 내용을 보여줍니다.)
It contains a single pragma directive that enables shader debugging:
(이 파일에는 셰이더 디버깅을 활성화하는 단일 #pragma
지시어가 포함되어 있습니다:)
// Comment out the following line to disable shader debugging // (다음 줄의 주석 처리('#')를 제거하면 hader debugging이 비활성화됩니다) #pragma enable_d3d11_debug_symbols
In each shader that you want to debug, add an #include_with_pragmas
directive that points to the include file.
(디버깅할 각 shader에 대해, 포함 파일을 가리키는 #include_with_pragmas
지시어를 추가하세요.)
Put the directive near the other #pragma
directives, like this:
(이 지시어를 다른 #pragma
지시어들 근처에 배치합니다.)
// Example pragma directives #pragma target 4.0 // Shader가 DirectX 11의 Shader 모델 4.0을 대상으로 한다는 것을 지정 #pragma vertex vert // vert라는 이름의 버텍스 Shader 함수를 사용하겠다고 선언 #pragma fragment frag // frag라는 이름의 프래그먼트 Shader 함수를 사용하겠다고 선언 // Replace path-to-include-file with the path to the include file // (지정된 경로의 포함 파일을 포함하며, 이 파일에 있는 #pragma 지시어도 함께 적용) // (경로 "path-to-include-file"는 실제 포함 파일의 경로로 대체해야 합니다.) #include_with_pragmas "path-to-include-file" // The rest of the HLSL code goes here ------------------------------ #include_with_pragmas "path-to-include-file" 지시어를 사용하면, 해당 경로에 있는 파일(path-to-include-file) 내부의 #pragma 지시어들을 여러 shader 파일에서 공유 여러 shader에서 공통으로 사용하는 #pragma 지시어를 한 곳에서 관리할 수 있게 됩니다.
Now, when you want to toggle shader debugging on and off for all shaders that use the include file, you only need to change a single line in the include file.
(이제 포함 파일을 사용하는 모든 shader에 대해 shader debugging을 켜고 끄고 싶을 때, 포함 파일의 한 줄만 변경하면 됩니다.)
When Unity recompiles the shaders, it includes the amended line.
(Unity가 셰이더를 다시 컴파일할 때, 수정된 줄이 포함됩니다.)
Note: If a shader file uses #include
to import a file that contains an #include_with_pragmas
directive, Unity ignores the #pragma
directives in the file the #include_with_pragmas
directive references.
(참고: shader 파일이 #include_with_pragmas
지시어를 포함하는 파일을 #include
로 가져오는 경우, Unity는 #include_with_pragmas
지시어가 참조하는 파일의 #pragma
지시어를 무시합니다)
Provide information to the shader compiler in HLSL
(HLSL의 shader compiler에 정보 제공)
https://docs.unity3d.com/2023.2/Documentation/Manual/SL-PragmaDirectives.html
In HLSL, you can use the following types of preprocessor directive to provide information to the shader compiler:
(HLSL에서 셰이더 컴파일러에 정보를 제공하기 위해 다음과 같은 전처리 지시어를 사용할 수 있습니다 🙂
#pragma
#define_for_platform_compiler
Pragma directives (Pragma 지시어)
#pragma
directives provide additional information to the shader compiler that isn’t covered by other types of preprocessor directive.
(#pragma
지시어는 다른 종류의 전처리 지시어로는 다룰 수 없는 추가적인 정보를 Shader Compiler에 제공합니다.)
You can put #pragma
directives anywhere in your HLSL code, but it is a common convention to put them at the start, like this:
(#pragma
지시어는 HLSL 코드의 어디에나 넣을 수 있지만, 일반적으로 코드의 시작 부분에 배치하는 것이 관례입니다. 다음과 같이 사용할 수 있습니다:)
#pragma target 3.0 // Shader가 DirectX 9의 Shader 모델 3.0을 대상으로 한다는 것을 지정 #pragma exclude_renderers vulkan // 특정 렌더링 API에서 Shader를 제외 / Vulkan 렌더링 API를 사용할 때는 컴파일되거나 실행되지 않음 #pragma vertex vert // vert라는 이름의 버텍스 Shader 함수를 사용하겠다고 선언 #pragma fragment frag // frag라는 이름의 프래그먼트 Shader 함수를 사용하겠다고 선언 // The rest of your HLSL code goes here // 나머지 HLSL 코드는 여기에 작성합니다
Limitations (제한 사항)
There are some limitations around the use of #pragma
directives:
(#pragma
지시어 사용에 몇 가지 제한 사항이 있습니다:)
- – You can use
#pragma
directives inside conditional (#if
) directives if the expression depends only on:
(#pragma
지시어는 조건부 지시어(#if
) 내에서 사용할 수 있지만, 조건식은 다음에만 의존해야 합니다:)- Any custom
#define
directives in your own code
(사용자 정의#define
지시어) - The following platform keywords :
(특정 플랫폼 키워드:)SHADER_API_MOBILE
,SHADER_API_DESKTOP
,UNITY_NO_RGBM
,UNITY_USE_NATIVE_HDR
,UNITY_FRAMEBUFFER_FETCH_AVAILABLE
,UNITY_NO_CUBEMAP_ARRAY
- The
UNITY_VERSION
macro
(UNITY_VERSION
매크로)
- Any custom
- – You can only use Unity-specific
#pragma
directives in.shader
files, and in files that you include with the#include_with_pragmas
directive.
( Unity-specific#pragma
지시어는.shader
파일과#include_with_pragmas
지시어로 포함된 파일에서만 사용할 수 있습니다.) - Unity does not support them in files that you include with the
#include
directive; the compiler ignores them.
(#include
지시어로 포함된 파일에서는 지원되지 않으며, 컴파일러는 이를 무시합니다.) - – You can only use standard HLSL
#pragma
directives in files that you include with the#include
directive.
(표준 HLSL#pragma
지시어는#include
지시어로 포함된 파일에서만 사용할 수 있습니다.)
Unity does not support them in.shader
files, or in files that you include with an#include_with_pragmas
directive; the compiler ignores them.
(.shader
파일이나#include_with_pragmas
지시어로 포함된 파일에서는 지원되지 않으며, 컴파일러는 이를 무시합니다.)
Note: If a shader file uses #include
to import a file that contains an #include_with_pragmas
directive, Unity ignores the #pragma
directives in the file the #include_with_pragmas
directive references.
(참고: 셰이더 파일이 #include
를 사용하여 #include_with_pragmas
지시어가 포함된 파일을 가져오는 경우,
Unity는 #include_with_pragmas
지시어가 참조하는 파일의 #pragma
지시어를 무시합니다.
=> #include
로 가져온 shader 파일이 #include_with_pragmas
지시어를 포함하는 경우,
Unity는 #include_with_pragmas
지시어가 참조하는 파일의 #pragma
지시어를 무시)
Standard pragma directives (표준 pragma 지시어)
Unity supports all #pragma directives that are part of standard HLSL, as long as these directives are in regular include files.
(Unity는 표준 HLSL의 모든 #pragma
지시어를 지원합니다, 이 지시어들은 일반적인 포함 파일에서 사용될 수 있습니다.)
For more information on these directives, see the HLSL documentation: pragma Directive.
(자세한 내용은 HLSL 문서의 pragma Directive
를 참조하세요.)
Surface Shaders (표면 셰이더)
If you are writing a Surface Shader, use this directive to tell the compiler which function to use as the surface function, and pass data to that function.
(Surface Shader를 작성할 때는 다음과 같은 지시어를 사용하여 컴파일러에 surface function과 그에 필요한 데이터를 전달할 수 있습니다:)
Statement | Function |
---|---|
#pragma surface <surface function> <lighting model> <optional parameters> | Compile the function with the given name as the surface shader, so that it works with the given lighting model. For more information, see Surface Shaders. (주어진 이름을 서피스 셰이더로 사용하여 주어진 조명 모델과 함께 작동하도록 함수를 컴파일합니다. 자세한 내용은 Surface Shaders를 참조하세요.) |
Shader stages (Shader 단계)
If you are writing a regular graphics shader, use these directives to tell the compiler which functions to use for different shader stages.
(일반 graphics shader를 작성할 때는 다음 지시어를 사용하여 컴파일러에 다양한 shader 단계에 사용할 함수를 알려줍니다.)
The #pragma vertex
and #pragma fragment
directives are required, but other stages are optional.
(#pragma vertex
와 #pragma fragment
지시어는 필수이며, 다른 단계는 선택적입니다.)
Statement | Function |
---|---|
#pragma vertex <name> | Compile the function with the given name as the vertex shader. (지정된 이름의 함수를 정점 셰이더로 컴파일합니다.) Replace <name> with the function name. ( <name> 을 함수 이름으로 교체하세요.)This directive is required in regular graphics shaders. (이 지시어는 일반 그래픽 셰이더에서 필수입니다.) |
#pragma fragment <name> | Compile the function with the given name as the fragment shader. (지정된 이름의 함수를 fragment shader로 컴파일합니다.) Replace <name> with the function name. ( <name> 을 함수 이름으로 교체하세요.)This directive is required in regular graphics shaders. (이 지시어는 일반 graphics shaders에서 필수입니다.) |
#pragma geometry <name> | Compile the function with the given name as the geometry shader. (지정된 이름의 함수를 기하학 셰이더로 컴파일합니다.) Replace <name> with the function name. (<name>을 함수 이름으로 교체하세요.) This option automatically turns on #pragma require geometry ; (이 옵션은 자동으로 #pragma require geometry 를 활성화합니다.)for more information, see Targeting shader models and GPU features in HLSL. (자세한 내용은 HLSL의 셰이더 모델 및 GPU 기능을 참조하세요.) Note: Metal does not support geometry shaders. (참고: Metal[애플]은 기하학 셰이더를 지원하지 않습니다.) |
#pragma hull <name> | Compile the function with the given name as the DirectX 11 hull shader. (지정된 이름의 함수를 DirectX 11 hull Shader로 컴파일합니다.) Replace <name> with the function name. (<name>을 함수 이름으로 교체하세요.) This automatically adds #pragma require tessellation ; (이 옵션은 자동으로 #pragma require tessellation을 활성화합니다.) for more information, see Targeting shader models and GPU features in HLSL. (자세한 내용은 HLSL의 셰이더 모델 및 GPU 기능을 참조하세요.) – hull Shader : shader 모델 5.0에서 도입된 기능으로, 복잡한 기하학적 세부사항을 동적으로 생성하는 데 사용 Hull Shader는 이 테셀레이션(일정한 형태의 도형들로 평면을 빈틈 없이 채우는 것.) 프로세스의 첫 번째 단계로, 입력된 기하학을 테셀레이션(세분화)할 수 있도록 정보를 제공 |
#pragma domain <name> | Compile the function with the given name as the DirectX 11 domain shader. (지정된 이름의 함수를 DirectX 11 Domain shader로 컴파일합니다.) Replace <name> with the function name. (<name>을 함수 이름으로 교체하세요.) This option automatically turns on #pragma require tessellation ; (이 옵션은 자동으로 #pragma require tessellation 을 활성화합니다.)for more information, see Targeting shader models and GPU features in HLSL. (자세한 내용은 HLSL의 셰이더 모델 및 GPU 기능을 참조하세요.) |
Shader variants and keywords (Shader 변형 및 키워드)
Use these directives to tell the shader compiler how to handle shader variants and keywords.
(다음 지시어를 사용하여 shader 컴파일러에 shader 변형 및 키워드를 처리하는 방법을 지시할 수 있습니다.)
For more information, see Declaring and using shader keywords in HLSL.
(자세한 내용은 HLSL의 셰이더 키워드 선언 및 사용을 참조하세요.)
Directive | Description |
---|---|
#pragma multi_compile <keywords> | Declares a collection of keywords. The compiler includes all of the keywords in the build. (여러 개의 키워드를 선언합니다. 컴파일러는 모든 키워드를 포함하여 빌드를 생성합니다.) You can use suffixes such as _local to set additional options.( _local 과 같은 접미사를 사용하여 추가 옵션을 설정할 수 있습니다.)For more information and a list of supported suffixes, see Declaring and using shader keywords in HLSL. (자세한 내용과 지원되는 접미사 목록은 HLSL의 shader 키워드 선언 및 사용을 참조하세요.) |
#pragma shader_feature <keywords> | Declares a collection of keywords. The compiler excludes unused keywords from the build. (여러 개의 키워드를 선언합니다. 컴파일러는 사용되지 않는 키워드를 빌드에서 제외합니다.) You can use suffixes such as _local to set additional options.( _local 과 같은 접미사를 사용하여 추가 옵션을 설정할 수 있습니다.)For more information and a list of supported suffixes, see Declaring and using shader keywords in HLSL. (자세한 내용과 지원되는 접미사 목록은 HLSL의 shader 키워드 선언 및 사용을 참조하세요.) |
#pragma hardware_tier_variants <values> | Built-in Render Pipeline only: Add keywords for graphics tiers when compiling for a given graphics API. (Built-in Render Pipeline에서만 사용됩니다. 주어진 그래픽 API를 위해 graphics tiers에 대한 키워드를 추가합니다.) For more information, see Graphics tiers. 그래픽 티어에 대한 자세한 내용은 Graphics tiers를 참조하세요. |
#pragma skip_variants <list of keywords> | Strip specified keywords. (지정된 키워드를 생략하여 빌드에서 제거합니다.) |
Graphics APIs
Use these directives to tell Unity to include or exclude code for a given graphics API.
(다음 지시어를 사용하여 Unity에 특정 그래픽 API에 대한 코드를 포함하거나 제외하도록 지시할 수 있습니다.)
Statement | Function |
---|---|
#pragma only_renderers <value> | Compile this shader program only for given graphics APIs. (이 셰이더 프로그램을 지정된 그래픽 API에 대해서만 컴파일합니다.) Replace <values> with a space-delimited list of valid values. ( <value> 를 유효한 값들의 공백으로 구분된 목록으로 교체하세요.)For more information and a list of valid values, see Targeting graphics APIs and platforms in HLSL. (자세한 내용과 유효한 값 목록은 HLSL의 그래픽 API 및 플랫폼 타겟팅을 참조하세요.) |
#pragma exclude_renderers <value> | Do not compile this shader program for given graphics APIs. (이 셰이더 프로그램을 지정된 그래픽 API에 대해 컴파일하지 않습니다.) Replace <value> with a space-delimited list of valid values. ( <value> 를 유효한 값들의 공백으로 구분된 목록으로 교체하세요.)For more information and a list of valid values, see Targeting graphics APIs and platforms in HLSL. (자세한 내용과 유효한 값 목록은 HLSL의 그래픽 API 및 플랫폼 타겟팅을 참조하세요.) |
Other pragma directives (기타 #pragma
지시어)
Statement | Function |
---|---|
#pragma instancing_options <options> | Enable GPU instancing in this shader, with given options. (주어진 옵션을 사용하여 이 셰이더에서 GPU 인스턴싱을 활성화합니다.) For more information, see GPU instancing (자세한 내용은 GPU instancing을 참조하세요.) |
#pragma once | Put this directive in a file to ensure that the compiler includes the file only once in a shader program. (이 지시어를 파일에 추가하면 컴파일러가 해당 파일을 셰이더 프로그램에서 한 번만 포함하도록 보장합니다.) Note: Unity only supports this directive when the Caching Shader Preprocessor is enabled. (참고: Unity는 이 지시어를 Caching Shader Preprocessor가 활성화된 경우에만 지원합니다.) |
#pragma enable_d3d11_debug_symbols | Generates shader debug symbols for supported graphics APIs, and disables optimizations for all graphics APIs. (지원되는 그래픽 API에 대해 셰이더 디버그 심볼을 생성하고, 모든 그래픽 API에 대해 최적화를 비활성화합니다.) Use this for debugging shader code in an external tool. (외부 도구에서 셰이더 코드를 디버깅할 때 사용합니다.) Unity generates debug symbols for Vulkan, DirectX 11 and 12, and supported console platforms. (Unity는 Vulkan, DirectX 11 및 12 및 지원되는 console platforms에 대한 debug symbols을 생성합니다.) Warning: Using this results in an increased file size and reduced shader performance. (이 지시어를 사용하면 파일 크기가 증가하고 셰이더 성능이 저하될 수 있습니다.) When you have finished debugging your shaders and you are ready to make a final build of your application, remove this line from your shader source code and recompile the shaders. (shader 디버깅을 완료하고 application을 최종 빌드할 준비가 되면 shader 소스 코드에서 이 줄을 제거하고 shader를 다시 컴파일합니다.) |
#pragma skip_optimizations <value> | Forces optimizations off for given graphics APIs. (주어진 그래픽 API에 대해 최적화를 강제로 비활성화합니다.) Replace <values> with a space-delimited list of valid values. ( <value> 를 유효한 값들의 공백으로 구분된 목록으로 교체하세요.)For a list of valid values, see Targeting graphics APIs and platforms in HLSL (유효한 값 목록은 HLSL의 그래픽 API 및 플랫폼 타겟팅을 참조하세요.) |
#pragma hlslcc_bytecode_disassembly | Embed disassembled HLSLcc bytecode into a translated shader. (번역된 셰이더에 disassembled HLSLcc 바이트코드를 포함합니다.) |
#pragma disable_fastmath | Enable precise IEEE 754 rules involving NaN handling. (NaN 처리와 관련된 IEEE 754 규칙을 정확히 따릅니다.) This currently only affects the Metal platform. (현재 Metal 플랫폼에서만 영향을 미칩니다.) |
#pragma editor_sync_compilation | Force synchronous compilation. This affects the Unity Editor only. (동기식 컴파일을 강제합니다. 이는 Unity Editor에만 영향을 미칩니다.) For more information, see Asynchronous Shader compilation. (자세한 내용은 Asynchronous(비동기) Shader 컴파일을 참조하세요.) |
#pragma enable_cbuffer | Emit cbuffer(name) when using CBUFFER_START(name) and CBUFFER_END macros from HLSLSupport even if the current platform does not support constant buffers.(현재 플랫폼이 상수 버퍼를 지원하지 않더라도 CBUFFER_START(name) 및 CBUFFER_END 매크로를 사용할 때 cbuffer(name) 을 생성합니다.) |
Use a define_for_platform_compiler directive
(#define_for_platform_compiler
지시어 사용하기)
Use a #define_for_platform_compiler
directive in your shader code to send a #define
directive to the shader compiler.
(shader 코드에서 #define_for_platform_compiler
지시어를 사용하여 shader 컴파일러에 #define
지시어를 전달할 수 있습니다.)
For example, #define_for_platform_compiler EXAMPLE_SYMBOL
sends a #define EXAMPLE_SYMBOL
directive to the shader compiler that defines a symbol called EXAMPLE_SYMBOL
.
(예를 들어, #define_for_platform_compiler EXAMPLE_SYMBOL
은 #define EXAMPLE_SYMBOL
지시어를 shader 컴파일러에 전달하여 EXAMPLE_SYMBOL
이라는 심볼을 정의합니다.)
Refer to external shader compiler documentation, for example Microsoft’s documentation on the FXC compiler, for more information about symbols that shader compilers use.
(이와 관련된 자세한 내용은 외부 셰이더 컴파일러 문서, 예를 들어 Microsoft의 FXC 컴파일러 문서를 참조하세요.)
The Unity preprocessor doesn’t use symbols you define with #define_for_platform_compiler
, so you can’t use the symbols in your own shader code.
(Unity 전처리기는 #define_for_platform_compiler
로 정의한 심볼을 사용하지 않습니다. 따라서, 이러한 심볼을 shader 코드에서 직접 사용할 수 없습니다.)
For example, in the above example, if you add shader code inside an #if (EXAMPLE_SYMBOL)
statement, the code won’t run.
(예를 들어, 위의 예제에서 #if (EXAMPLE_SYMBOL)
구문 안에 셰이더 코드를 추가하더라도 그 코드는 실행되지 않습니다.)
Targeting shader models and GPU features in HLSL
(HLSL의 셰이더 모델 및 GPU 기능 타겟팅)
https://docs.unity3d.com/2022.3/Documentation/Manual/SL-ShaderCompileTargets.html
You can use #pragma
directives to indicate that a shader requires certain GPU features.
(#pragma 지시어를 사용하여 Shader가 특정 GPU 기능을 필요로 한다는 것을 명시할 수 있습니다.)
At runtime, Unity uses this information to determine whether a shader program is compatible with the current hardware.
(Unity는 런타임 시 이 정보를 사용하여 Shader 프로그램이 현재 하드웨어와 호환되는지 여부를 판단합니다.)
You can specify individual GPU features with the #pragma require
directive, or specify a shader model with the #pragma target
directive.
(#pragma require 지시어로 개별 GPU 기능을 지정하거나, #pragma target 지시어로 Shader 모델을 지정할 수 있습니다.)
A shader model is a shorthand for a group of GPU features; internally, it is the same as a #pragmarequire
directive with the same list of features.
(Shader 모델은 여러 GPU 기능을 하나로 묶은 단축어로, 내부적으로 동일한 기능 목록을 가진 #pragma require 지시어와 동일합니다.)
It is important to correctly describe the GPU features that your shader requires.
(Shader가 필요로 하는 GPU 기능을 정확히 기술하는 것이 중요합니다.)
If your shader uses features that are not included in the list of requirements, this can result in either compile time errors, or in devices failing to support shaders at runtime.
(Shader에서 사용한 기능이 요구 사항 목록에 포함되지 않은 경우, 컴파일 시 오류가 발생하거나 런타임 시 디바이스에서 Shader를 지원하지 못하는 상황이 발생할 수 있습니다.)
Default behavior (기본 동작)
By default, Unity compiles shaders with #pragma require derivatives
, which corresponds to #pragma target 2.5
.
(Unity는 기본적으로 #pragma require derivatives로 Shader를 컴파일하며, 이는 #pragma target 2.5에 해당합니다.)
Special requirements for shader stages (shader 단계에 대한 특별 요구 사항)
If your shader defines certain shader stages, Unity automatically adds items to the list of requirements.
(Shader가 특정 Shader 단계를 정의하는 경우, Unity는 자동으로 요구 사항 목록에 항목을 추가합니다.)
- – If a shader defines a geometry stage (with
#pragma geometry
), Unity automatically addsgeometry
to the list of requirements.
(#pragma geometry), Unity는 자동으로 요구 사항 목록에 geometry를 추가합니다.) - – If a shader defines a tessellation stage (with
#pragma hull
or#pragma domain
), Unity automatically addstessellation
to the list of requirements.
(#pragma hull 또는 #pragma domain), Unity는 자동으로 요구 사항 목록에 tessellation을 추가합니다.)
If the list of requirements (or the equivalent target value) does not already include these values, Unity displays a warning message when it compiles the shader, to indicate that it has added these requirements.
(요구 사항 목록에 이러한 값들이 이미 포함되어 있지 않다면, Unity는 Shader를 컴파일할 때 이러한 요구 사항이 추가되었음을 알리는 경고 메시지를 표시합니다.)
To avoid seeing this warning message, explicitly add the requirements or use an appropriate target value in your code.
(이 경고 메시지를 피하려면 코드에서 명시적으로 요구 사항을 추가하거나 적절한 target 값을 사용해야 합니다.)
Specifying GPU features or a shader model (GPU 기능 또는 셰이더 모델 지정)
To specify required features, use the #pragma require
directive, followed by a list of space-delimited values. For example:
(필요한 기능을 지정하려면, #pragma require 지시어를 사용하고 공백으로 구분된 값들의 목록을 나열합니다. 예를 들어서:)
#pragma require integers mrt8 // integers: Shader에서 정수 연산을 지원하는 GPU를 요구 // 이는 정수 기반의 계산이나 비트 연산을 수행하는 Shader에서 필수 // mrt8: 최대 8개의 Multiple Render Targets (MRTs)을 지원하는 GPU를 요구 // mrt는 Shader가 한 번의 렌더 패스에서 여러 개의 렌더 타겟에 출력할 수 있도록 하는 기능
You can also use the #pragma require
directive followed by a colon and a list of space-delimited shader keywords.
(#pragma require 지시어 뒤에 콜론과 공백으로 구분된 Shader 키워드 목록을 추가할 수도 있습니다.)
This means that the requirement applies only to variants that are used when any of the given keywords are enabled. For example:
(이는 지정된 키워드가 활성화될 때 사용되는 변형에만 해당 요구 사항이 적용됨을 의미합니다. 예를 들어)
#pragma require integers mrt8 : EXAMPLE_KEYWORD OTHER_EXAMPLE_KEYWORD
You can use multiple #pragma require
lines.
(#pragma require 지시어를 여러 줄 사용할 수 있습니다.)
In this example, the shader requires integers
in all cases, and mrt8
if EXAMPLE_KEYWORD is enabled.
(이 예제에서는 Shader가 모든 경우에 integers를 필요로 하며, EXAMPLE_KEYWORD가 활성화된 경우 mrt8도 필요로 합니다.)
#pragma require integers #pragma require integers mrt8 : EXAMPLE_KEYWORD
To specify a shader model, use #pragma target
directive. For example:
(Shader 모델을 지정하려면, #pragma target 지시어를 사용하십시오.)
#pragma target 4.0
You can also use the #pragma target
directive followed by a list of space-delimited shader keywords.
(#pragma target 지시어 뒤에 공백으로 구분된 Shader 키워드 목록을 추가할 수도 있습니다.)
This means that the requirement applies only to variants that are used when any of the given keywords are enabled. For example:
(이는 지정된 키워드가 활성화될 때 사용되는 변형에만 해당 요구 사항이 적용됨을 의미합니다. 를 들어서)
#pragma target 4.0 EXAMPLE_KEYWORD OTHER_EXAMPLE_KEYWORD
Note: The syntax for specifying keywords for #pragma require
and #pragma target
is slightly different.
(참고: #pragma require와 #pragma target에 대한 키워드를 지정하는 문법은 약간 다릅니다.)
When you specify keywords for #pragma require
, you use a colon.
(#pragma require에 키워드를 지정할 때는 콜론(:)을 사용합니다.)
When you specify keywords for #pragma target
, you do not use a colon.
(#pragma target에 키워드를 지정할 때는 콜론을 사용하지 않습니다.)
List of ‘#pragma target’ values (#pragma target
값 목록)
Here is the list of shader models that Unity uses, and the combination of #pragma require
values that each corresponds to.
(다음은 Unity에서 사용하는 Shader 모델 목록과 각각에 해당하는 #pragma require
값의 조합입니다.)
Note: Unity’s shader models are similar to DirectX shader models and OpenGL version requirements, but they do not correspond exactly.
(참고: Unity의 Shader 모델은 DirectX Shader 모델 및 OpenGL 버전 요구 사항과 유사하지만, 정확히 일치하지는 않습니다.)
Read the descriptions carefully to ensure that you understand the differences.
(차이를 이해하려면 설명을 주의 깊게 읽으십시오.)
Value | Description | Support | Equivalent #pragma require values |
---|---|---|---|
2.0 | Equivalent to DirectX shader model 2.0. (DirectX Shader 모델 2.0과 동일합니다.) Limited amount of arithmetic and texture instructions; (제한된 산술 및 텍스처 명령어;) 8 interpolators; no vertex texture sampling; no derivatives in fragment shaders; no explicit LOD texture sampling. (8개의 보간자; 버텍스 텍스처 샘플링 없음; 프래그먼트 Shader에서 미분 없음; 명시적인 LOD 텍스처 샘플링 없음.)) | Works on all platforms supported by Unity. (Unity가 지원하는 모든 플랫폼에서 작동합니다.) | N/A |
2.5 | Almost the same as 3.0, but with only 8 interpolators, and no explicit LOD texture sampling. (Shader 모델 3.0과 거의 동일하지만, 8개의 보간자만 사용하며 명시적인 LOD 텍스처 샘플링이 없습니다.) | DirectX 11 feature level 9+ OpenGL 3.2+ OpenGL ES 2.0 Vulkan Metal | derivatives |
3.0 | Equivalent to DirectX shader model 3.0. (DirectX Shader 모델 3.0과 동일합니다.) | DirectX 11 feature level 10 + OpenGL 3.2+ OpenGL ES 3.0+ Vulkan Metal Might work on some OpenGL ES 2.0 devices, depending on driver extensions and features. (드라이버 확장 및 기능에 따라 일부 OpenGL ES 2.0 디바이스에서 작동할 수 있습니다.) | Everything in 2.5 , plus:(2.5에 추가적으로:) interpolators10 samplelod fragcoord |
3.5 | Equivalent to OpenGL ES 3.0. (OpenGL ES 3.0과 동일합니다.) | DirectX 11 feature level 10+ OpenGL 3.2+ OpenGL ES 3+ Vulkan Metal | Everything in 3.0 , plus:(3.0에 추가적으로:) interpolators15 mrt4 integers 2darray instancing |
4.0 | Equivalent to DirectX shader model 4.0, but without the requirement to support 8 MRTs. (DirectX Shader 모델 4.0과 동일하지만, 8 MRT 지원 요구 사항은 없습니다.) | DirectX 11 feature level 10+ Desktop OpenGL 3.x OpenGL ES 3.1 OpenGL 3.2+ OpenGL ES 3.1 + Android Extension Pack (AEP) Vulkan Metal (if no geometry stage is defined) (Metal (geometry 단계가 정의되지 않은 경우)) | Everything in 3.5 , plus:(3.5에 추가적으로:) geometry |
gl4.1 | Equivalent to OpenGL 4.1 (OpenGL 4.1과 동일합니다.) | Desktop OpenGL 4.1 (데스크톱 OpenGL 4.1) Shader model 4.0 + tessellation to match macOS 10.9 capabilities. (Shader 모델 4.0에 tessellation이 추가되어 macOS 10.9 기능과 일치합니다.) | Everything in 4.0 , plus:(4.0에 추가적으로:) cubearray tesshw tessellation msaatex |
4.5 | Equivalent to OpenGL ES 3.1. (OpenGL ES 3.1과 동일합니다.) | DirectX 11 feature level 11+ OpenGL 4.3+ OpenGL ES 3.1 Vulkan Metal | Everything in 3.5 , plus:(3.5에 추가적으로:) compute randomwrite msaatex |
4.6 | Equivalent to OpenGL 4.1. (OpenGL 4.1과 동일합니다.) This is the highest OpenGL level supported on a Mac. 이것이 Mac에서 지원되는 최고 OpenGL 수준입니다.) | DirectX 11 feature level 11+ OpenGL 4.1+ OpenGL ES 3.1 + AEP Vulkan Metal (if no geometry stage is defined, and no hull or domain stage is defined) (Metal (geometry 단계나 hull 또는 domain 단계가 정의되지 않은 경우)) | Everything in 4.0 , plus:(4.0에 추가적으로:) cubearray tesshw tessellation msaatex |
5.0 | Equivalent to DirectX shader model 5.0, but without the requirement to support 32 interpolators or cubemap arrays. (DirectX Shader 모델 5.0과 동일하지만, 32개의 보간자나 cubemap 배열 지원 요구 사항은 없습니다.) | DirectX 11 feature level 11+ Desktop OpenGL >= 4.2 OpenGL 4.3+ OpenGL ES 3.1 + AEP Vulkan Metal (if no geometry stage is defined, and no hull or domain stage is defined) (Metal (geometry 단계나 hull 또는 domain 단계가 정의되지 않은 경우)) | Everything in 4.0 , plus:(4.0에 추가적으로:) compute randomwrite msaatex tesshw tessellation |
For information on shader model support for console platforms, see the platform-specific documentation.
(콘솔 플랫폼의 Shader 모델 지원에 대한 정보는 플랫폼별 문서를 참조하십시오.)
Notes:
- – In the DirectX definitions, shader model 4.0 includes
mrt8
, and shader model 5.0 includesinterpolators32
andcubearray
.
(DirectX 정의에서 Shader 모델 4.0은 mrt8을 포함하고, Shader 모델 5.0은 interpolators32와 cubearray를 포함합니다.) - Unity does not include these, for broader compatibility. To require these features, use an explicit
#pragma require
directive.
(Unity는 더 넓은 호환성을 위해 이를 포함하지 않습니다. 이러한 기능이 필요할 경우, 명시적으로#pragma require
지시어를 사용하십시오.) - – If you use a target that requires
geometry
but your shader does not define a geometry stage, Unity removesgeometry
from the list of requirements at compile time.
(geometry가 필요한 target을 사용하였으나 Shader가 geometry 단계를 정의하지 않은 경우, Unity는 컴파일 시 요구 사항 목록에서 geometry를 제거합니다.) - – If you use a target that requires
tessellation
but your shader does not define a hull or domain stage, Unity removestessellation
from the list of requirements at compile time.
(tessellation이 필요한 target을 사용하였으나 Shader가 hull 또는 domain 단계를 정의하지 않은 경우, Unity는 컴파일 시 tessellation을 요구 사항 목록에서 제거합니다.)
List of ‘#pragma require’ values (‘#pragma required’ 값 목록)
Here are all the valid values for the #pragma require
directive.
(다음은 #pragma require
지시어에 대한 모든 유효한 값들입니다.)
Value | Description |
---|---|
interpolators10 | At least 10 vertex-to-fragment interpolators (“varyings”) are available. (최소 10개의 버텍스-프래그먼트 보간자(“varyings”)가 제공됩니다.) |
interpolators15 | At least 15 vertex-to-fragment interpolators (“varyings”) are available. (최소 15개의 버텍스-프래그먼트 보간자(“varyings”)가 제공됩니다.) Note: Internally, this also automatically adds integers to the list of requirements.(참고: 내부적으로, 이는 자동으로 integers 를 요구 사항 목록에 추가합니다.) |
interpolators32 | At least 32 vertex-to-fragment interpolators (“varyings”) are available. (최소 32개의 버텍스-프래그먼트 보간자(“varyings”)가 제공됩니다.) |
integers | Integers are a supported data type, including bit/shift operations. (정수는 비트/시프트 연산을 포함한 지원 데이터 타입입니다.) Note: Internally, this also automatically adds interpolators15 to the list of requirements.(참고: 내부적으로, 이는 자동으로 interpolators15 를 요구 사항 목록에 추가합니다.) |
mrt4 | At least 4 render targets are supported. (최소 4개의 렌더 타겟이 지원됩니다.) |
mrt8 | At least 8 render targets are supported. (최소 8개의 렌더 타겟이 지원됩니다.) |
derivatives | Pixel shader derivative instructions (ddx/ddy) are supported. (Pixel shader 미분 명령(ddx/ddy)이 지원됩니다.) |
samplelod | Explicit texture LOD sampling (tex2Dlod / SampleLevel) is supported. (명시적인 텍스처 LOD 샘플링(tex2Dlod / SampleLevel)이 지원됩니다.) |
fragcoord | Pixel location (XY on screen, ZW depth in clip space) input in pixel shader is supported. (Pixel shader에서 Pixel 위치(화면상의 XY, 클립 공간에서의 ZW 깊이) 입력이 지원됩니다.) |
2darray | 2D texture arrays are a supported data type. (2D 텍스처 배열이 지원되는 데이터 타입입니다.) |
cubearray | Cubemap arrays are a supported data type. (Cubemap 배열이 지원되는 데이터 타입입니다.) |
instancing | SV_InstanceID input system value is supported. (SV_InstanceID 입력 시스템 값이 지원됩니다.) |
geometry | Geometry shader stages are supported. (Geometry shader 단계가 지원됩니다.) |
compute | Compute shaders, structured buffers, and atomic operations are supported. (Compute shader, 구조화된 버퍼, 원자 연산이 지원됩니다.) |
randomwrite or uav | “Random write” (UAV) textures are supported. (“랜덤 쓰기”(UAV) 텍스처가 지원됩니다.) |
tesshw | Hardware tessellation is supported, but not necessarily tessellation (hull/domain) shader stages. (하드웨어 테셀레이션이 지원되지만, 테셀레이션(hull/domain) shader 단계는 필수적으로 지원되지는 않습니다. ) For example, Metal supports tessellation, but not hull or domain stages. (예를 들어, Metal은 테셀레이션을 지원하지만 hull 또는 domain 단계를 지원하지 않습니다.) |
tessellation | Tessellation (hull/domain) shader stages are supported. (테셀레이션(hull/domain) shader 단계가 지원됩니다.) |
msaatex | The ability to access multi-sampled textures (Texture2DMS in HLSL) is supported. (멀티 샘플링된 텍스처(Texture2DMS in HLSL)에 접근할 수 있는 기능이 지원됩니다.) |
sparsetex | Sparse textures with residency info (“Tier2” support in DirectX terms; CheckAccessFullyMapped HLSL function).(레지던시 정보가 포함된 Sparse 텍스처(DirectX 용어로 “Tier2” 지원; HLSL 함수 CheckAccessFullyMapped ).) |
framebufferfetch or fbfetch | Framebuffer fetch (the ability to read input pixel color in the pixel shader) is supported. (프레임버퍼 패치(pixel shader에서 입력 픽셀 색상을 읽을 수 있는 기능)가 지원됩니다.) |
setrtarrayindexfromanyshader | Setting the render target array index from any shader stage (not just the geometry shader stage) is supported. (Geometry shader 단계뿐만 아니라 모든 shader 단계에서 렌더 타겟 배열 인덱스를 설정할 수 있는 기능이 지원됩니다.) |
inlineraytracing | Inline ray tracing is supported, so you can generate ray queries in the rasterization and compute stages of a shader. (인라인 레이 트레이싱이 지원되어, 쉐이더의 래스터화 및 컴퓨팅 단계에서 레이 쿼리를 생성할 수 있습니다.) Refer to SystemInfo.supportsInlineRayTracing for more information. (자세한 내용은 SystemInfo.supportsInlineRayTracing 을 참조하세요.) |
Targeting graphics APIs and platforms in HLSL
(HLSL에서 그래픽 API 및 플랫폼 타겟팅)
Some #pragma
directives take parameters that allow you to target specific graphics APIs and platforms.
(일부 #pragma
지시어는 특정 그래픽 API 및 플랫폼을 타겟팅할 수 있는 매개변수를 받습니다.)
This page contains information on using those directives, and provides a list of valid parameter values.
(이 페이지는 이러한 지시어 사용에 대한 정보를 제공하며, 유효한 매개변수 값 목록을 제공합니다.)
Including or excluding graphics APIs
(그래픽 API 포함 또는 제외)
By default, Unity compiles all shader programs for each graphics API in the list for the current build target.
(기본적으로 Unity는 현재 빌드 타겟의 목록에 있는 각 그래픽 API에 대해 모든 shader 프로그램을 컴파일합니다.)
Sometimes, you might want to compile certain shader programs only for certain graphics APIs; for example, if you use features that are not supported on all platforms.
(때로는 특정 그래픽 API에만 Shader 프로그램을 컴파일하고 싶을 수 있습니다. 예를 들어, 모든 플랫폼에서 지원되지 않는 기능을 사용할 경우입니다.)
To compile a shader program only for given APIs, use the #pragma only_renderers
directive. You can pass multiple values, space delimited.
(특정 API에 대해서만 shader 프로그램을 컴파일하려면 #pragma only_renderers
지시어를 사용하십시오. 여러 값을 공백으로 구분하여 전달할 수 있습니다.)
This example demonstrates how to compile shaders only for Metal and Vulkan:
(다음 예는 Metal 및 Vulkan에 대해서만 shader를 컴파일하는 방법을 보여줍니다.)
#pragma only_renderers metal vulkan
To exclude shader code from compilation by given compilers, use the #pragma exclude_renderers
directive.
(특정 컴파일러에서 쉐이더 코드를 컴파일하지 않으려면 #pragma exclude_renderers
지시어를 사용하십시오. )
You can pass multiple values, space delimited.
(여러 값을 공백으로 구분하여 전달할 수 있습니다.)
This example demonstrates how to exclude a shader from compilation for Metal and Vulkan:
(다음 예는 Metal 및 Vulkan에 대한 shader 컴파일을 제외하는 방법을 보여줍니다.)
#pragma exclude_renderers metal vulkan
Generating shader variants for graphics tiers for a given graphics API
(주어진 그래픽 API에 대한 그래픽 티어의 shdaer 변형 생성)
In the Built-in Render Pipeline, Unity automatically generates shader variants that correspond to graphics tiers under certain conditions.
(Built-in Render Pipeline에서 Unity는 특정 조건 하에 graphics tiers에 해당하는 shader variants을 자동으로 생성합니다.)
You can also force Unity to generate these variants, if required.
(필요시 Unity에 강제로 이러한 변형을 생성하게 할 수 있습니다.)
To do this, use the #pragma hardware_tier_variants
preprocessor directive and specify the graphics APIs for which you want to generate tier shader variants.
(이를 위해 #pragma hardware_tier_variants
전처리 지시어를 사용하고 tier shader 변형을 생성하려는 그래픽 API를 지정하십시오.)
For example, this instructs Unity to compile tier shader variants for Metal:
(예를 들어, 이는 Unity가 Metal에 대한 tier shader 변형을 컴파일하도록 지시합니다.)
#pragma hardware_tier_variants metal
List of valid parameter values (유효한 매개변수 값 목록)
Supported values are:
(지원되는 값은 다음과 같습니다:)
Value | Description |
---|---|
d3d11 | DirectX 11 feature level 10 and above, DirectX 12 |
gles3 | OpenGL ES 3.x, WebGL 2.0 |
ps4 | PlayStation 4 |
xboxone | Xbox One and GameCore, DirectX 11 and DirectX 12 |
metal | iOS/Mac Metal |
glcore | OpenGL 3.x, OpenGL 4.x |
vulkan | Vulkan |
switch | Nintendo Switch |
ps5 | PlayStation 5 |
Declaring and using shader keywords in HLSL
(HLSL에서 쉐이더 키워드 선언 및 사용)
You can declare shader keywords so shaders behave differently when you enable or disable the keywords.
(shader키워드를 선언하여 키워드를 활성화하거나 비활성화할 때 shader가 다르게 동작하도록 할 수 있습니다.)
You can declare shader keywords in regular graphics shaders including surface shaders, and compute shaders.
(regular graphics shaders 및 surface shaders, compute shaders에서 shader 키워드를 선언할 수 있습니다.)
Declare shader keywords using pragma
(pragma를 사용한 shader 키워드 선언)
To declare shader keywords, use a #pragma
directive in the HLSL code. For example:
(shader 키워드를 선언하려면 HLSL 코드에서 #pragma
지시어를 사용하십시오. 예를 들어:)
#pragma shader_feature REFLECTION_TYPE1 REFLECTION_TYPE2 REFLECTION_TYPE3
You can use one of the following shader directives:
(다음 쉐이더 지시어 중 하나를 사용할 수 있습니다:)
Shader directive | Branching type | Shader variants Unity creates |
---|---|---|
shader_feature | Static branching | Variants for keyword combinations you enable at build time 빌드 시점에서 활성화한 키워드 조합에 대한 변형을 생성합니다. |
multi_compile | Static branching | Variants for every possible combination of keywords 가능한 모든 키워드 조합에 대한 변형을 생성합니다. |
dynamic_branch | Dynamic branching | No variants 변형을 생성하지 않습니다. |
Read more about when to use which shader directive.
(어떤 shader 지시어를 사용할지에 대한 추가 정보는 따로 참조하세요.)
See shader keyword limits.
(shader 키워드 제한 사항을 참조하세요.)
How sets of keywords work (키워드 집합이 작동하는 방식)
The keywords in a single #pragma
statement are together called a ‘set’.
(단일 #pragma
문에 포함된 키워드는 ‘집합’이라고 합니다.)
You can enable or disable multiple keywords in a set at the same time.
( 한 번에 여러 키워드를 활성화하거나 비활성화할 수 있습니다.)
For example, to declare a set of three keywords:
(예를 들어, 세 개의 키워드 집합을 선언하려면:)
#pragma shader_feature REFLECTION_TYPE1 REFLECTION_TYPE2 REFLECTION_TYPE3
You can declare multiple sets of keywords in a single shader. For example, to create 2 sets:
(단일 shader에서 여러 키워드 집합을 선언할 수 있습니다. 예를 들어, 두 개의 집합을 만들려면:)
#pragma shader_feature REFLECTION_TYPE1 REFLECTION_TYPE2 REFLECTION_TYPE3 #pragma shader_feature RED GREEN BLUE WHITE
You can’t do the following:
(다음은 할 수 없습니다:)
- – Include two keywords with the same name in one set.
(한 집합에 동일한 이름의 키워드를 포함할 수 없습니다.) - – Include duplicate keyword sets in one shader.
(하나의 shader에서 중복된 키워드 집합을 포함할 수 없습니다.) - – Declare a keyword as both
dynamic_branch
andshader_feature
ormulti_compile
– Unity usesdynamic_branch
if you do this.
(dynamic_branch
와shader_feature
또는multi_compile
을 동시에 선언할 수 없습니다. Unity는 이 경우dynamic_branch
를 사용합니다.)
Make shader behavior conditional (쉐이더 동작을 조건부로 설정)
To mark parts of your shader code conditional based on whether you enable or disable a shader keyword, use an HLSL if statement.
(shader 키워드의 활성화 여부에 따라 shader 코드의 일부를 조건부로 설정하려면 HLSL의 if
문을 사용하십시오.)
For example:
(예를 들어:)
#pragma multi_compile QUALITY_LOW QUALITY_MED QUALITY_HIGH if (QUALITY_LOW) { // code for low quality setting // 저품질 설정에 대한 코드 }
You can enable and disable keywords using the Inspector or C# scripting.
(Inspector나 C# 스크립팅을 사용하여 키워드를 활성화하거나 비활성화할 수 있습니다.)
What Unity does with your shader code depends on which shader directive you use.
(Unity가 shader 코드에서 하는 작업은 사용하는 shader 지시어에 따라 다릅니다.)
If you use dynamic_branch
, Unity creates a uniform Boolean variable for each keyword.
(만약 dynamic_branch
를 사용하면, Unity는 각 키워드에 대해 uniform Boolean 변수를 생성합니다.)
When you enable a keyword, Unity sets the Boolean for that variable to true
, and your GPU switches to using the code in the if
statement for that keyword.
(키워드를 활성화하면 Unity는 해당 변수를 true로 설정하고 GPU가 해당 키워드의 if
문 코드를 사용하도록 전환합니다.)
This is dynamic branching.
(이것이 동적 분기입니다.)
If you use shader_feature
or multi_compile
, Unity creates separate shader variants for each keyword state.
(shader_feature
나 multi_compile
을 사용하는 경우 Unity는 각 키워드 상태에 대해 별도의 shader variants을 생성합니다.)
Each variant contains the code from an if
branch for that keyword.
(각 변형은 해당 키워드의 if
분기 코드를 포함합니다.)
When you enable a keyword, Unity sends the matching variant to your GPU. This is static branching.
(키워드를 활성화하면 Unity는 해당 variant을 GPU로 보냅니다. 이것이 정적 분기입니다.)
Read more about when to use which shader directive.
(어떤 shader 지시어를 사용할지에 대한 추가 정보는 따로 참조하세요.)
Use other statements to make shader behavior conditional
(다른 명령문을 사용하여 Shader 동작을 조건부로 설정)
You can also use the following HLSL statements to create conditional code:
(다음 HLSL 명령문을 사용하여 조건부 코드를 만들 수 있습니다:)
Using these instead of if
makes it more difficult to change the #pragma
keyword directive later.
(이 명령문들을 if
대신 사용하는 것은 나중에 #pragma
키워드 지시어를 변경하기 어렵게 만듭니다.)
For example, if you need to reduce the number of shader variants, it’s more difficult to change multi_compile
to shader_feature
.
(예를 들어, shader 변형의 수를 줄여야 할 경우 multi_compile
을 shader_feature
로 변경하기가 더 어려워집니다.)
Make keywords local (키워드를 로컬로 만들기)
Keywords are global by default.
(키워드는 기본적으로 전역입니다.)
Add _local
to the shader directive to make the keywords local.
(키워드를 로컬로 만들려면 쉐이더 지시어에 _local
을 추가하십시오.)
If you enable or disable a global keyword, you don’t affect the state of local keywords with the same name.
(전역 키워드를 활성화하거나 비활성화해도 동일한 이름을 가진 로컬 키워드의 상태에 영향을 미치지 않습니다.)
For example:
(예를 들어:)
#pragma shader_feature_local QUALITY_LOW QUALITY_MED QUALITY_HIGH
Restrict keywords to shader stages (키워드를 쉐이더 단계로 제한)
When you declare a keyword, Unity assumes all stages of the shader contain conditional code for that keyword.
(키워드를 선언할 때 Unity는 쉐이더의 모든 단계에 대해 해당 키워드에 대한 조건부 코드가 포함된 것으로 간주합니다.)
You can add the following suffixes to indicate that only certain stages contain conditional code for a keyword.
(다음 접미사를 추가하여 특정 단계에만 조건부 코드가 포함되어 있음을 나타낼 수 있습니다.)
This helps Unity strip unneeded shader variants.
(이는 Unity가 불필요한 쉐이더 변형을 제거하는 데 도움이 됩니다.)
_vertex
_fragment
_hull
_domain
_geometry
_raytracing
For example, use #pragma shader_feature_fragment RED GREEN BLUE
to indicate that you use the 3 keywords to create conditional code in the fragment stage only.
(예를 들어, #pragma shader_feature_fragment RED GREEN BLUE
를 사용하여 세 가지 키워드를 사용해 fragment 단계에서만 조건부 코드를 생성함을 나타냅니다.)
You can’t add these suffixes to #pragma dynamic_branch
because dynamic_branch
doesn’t create variants.
(dynamic_branch
는 변형을 생성하지 않기 때문에 이 접미사를 추가할 수 없습니다.)
These suffixes may behave differently or have no effect depending on the graphics API:
(이 접미사는 그래픽 API에 따라 다르게 작동하거나 아무런 효과가 없을 수 있습니다:)
- – The suffixes have no effect on OpenGL, OpenGL ES or Vulkan.
(이 접미사는 OpenGL, OpenGL ES 또는 Vulkan에서는 효과가 없습니다.) - – The
_geometry
and_raytracing
suffixes have no effect on Metal, and Metal treats_vertex
,_hull
and_domain
as a single stage.
(_geometry
및_raytracing
접미사는 Metal에서는 효과가 없으며, Metal은_vertex
,_hull
,_domain
을 하나의 단계로 처리합니다.)
Restrict keywords to shader models and GPU features
(키워드를 shader 모델과 GPU 기능으로 제한)
You can add keywords to #pragma require
and #pragma target
directives, so conditional code only runs if the current hardware uses a particular shader model or GPU feature.
(#pragma require
및 #pragma target
지시어에 키워드를 추가하여 현재 하드웨어가 특정 쉐이더 모델 또는 GPU 기능을 사용하는 경우에만 조건부 코드가 실행되도록 할 수 있습니다.)
For more information, see Targeting shader models and GPU features in HLSL.
(자세한 내용은 HLSL에서 쉐이더 모델 및 GPU 기능 타겟팅을 참조하십시오.)
Create a shader variant for disabled keywords
(비활성화된 키워드에 대한 shader 변형 생성)
If you use shader_feature
to create a single keyword, Unity automatically creates a second variant for when the feature is disabled.
(단일 키워드를 생성하기 위해 shader_feature
를 사용하는 경우, Unity는 해당 기능이 비활성화될 때 두 번째 변형을 자동으로 생성합니다.)
This helps reduce the number of keywords you need to enable and disable.
(이는 활성화 및 비활성화해야 하는 키워드 수를 줄이는 데 도움이 됩니다.)
For example, the following code creates 2 variants:
(예를 들어, 다음 코드는 2개의 변형을 생성합니다:)
#pragma shader_feature EXAMPLE_ON
If you use multi_compile
, or you use shader_feature
to create a set of two or more keywords, you can use _
when you declare a keyword set.
(multi_compile
을 사용하거나 shader_feature
를 사용하여 두 개 이상의 키워드 집합을 생성하는 경우, 키워드 집합을 선언할 때 _
를 사용할 수 있습니다.)
Unity creates a shader variant for situations when all keywords in that set are disabled.
(Unity는 해당 집합의 모든 키워드가 비활성화된 상황에 대한 shader변형을 생성합니다.)
#pragma multi_compile _ EXAMPLE_ON #pragma shader_feature _ RED GREEN BLUE WHITE
Use shortcuts to create keyword sets
(단축어를 사용하여 키워드 집합 생성)
You can use Unity shader directive shortcuts to create sets of shader variants.
(Unity shader 지시어 단축어를 사용하여 shader 변형 집합을 생성할 수 있습니다.)
The following example explains how to add SHADOWS_DEPTH
and SHADOWS_CUBE
variants:
(다음 예는 SHADOWS_DEPTH
및 SHADOWS_CUBE
변형을 추가하는 방법을 설명합니다:)
#pragma multi_compile_shadowcaster
You can remove keywords you don’t need using skip_variants
.
(skip_variants
를 사용하여 필요하지 않은 키워드를 제거할 수 있습니다.)
For example, use the following to remove POINT
and POINT_COOKIES
variants when Unity generates variants from multi_compile_fwdadd
.
(예를 들어, Unity가 multi_compile_fwdadd
에서 변형을 생성할 때 POINT
및 POINT_COOKIES
변형을 제거하려면 다음을 사용하십시오:)
#pragma multi_compile_fwdadd #pragma skip_variants POINT POINT_COOKIE
The following shortcuts relate to light, shadow and lightmapping in the Built-in Render Pipeline :
(다음 단축어는 내장 렌더 파이프라인의 조명, 그림자 및 라이트매핑과 관련이 있습니다:)
- multi_compile_fwdbase
adds this set of keywords:
(multi_compile_fwdbase는 다음과 같은 키워드 집합을 추가합니다:)
DIRECTIONAL LIGHTMAP_ON DIRLIGHTMAP_COMBINED DYNAMICLIGHTMAP_ON SHADOWS_SCREEN SHADOWS_SHADOWMASK LIGHTMAP_SHADOW_MIXING LIGHTPROBE_SH.
These variants are needed by PassType.ForwardBase.
이 변형들은PassType.ForwardBase
에 필요합니다.- multi_compile_fwdbasealpha
adds this set of keywords:
(multi_compile_fwdadd
는 다음과 같은 키워드 집합을 추가합니다:)
DIRECTIONAL LIGHTMAP_ON DIRLIGHTMAP_COMBINED DYNAMICLIGHTMAP_ON LIGHTMAP_SHADOW_MIXING VERTEXLIGHT_ON LIGHTPROBE_SH.
These variants are needed by PassType.ForwardBase.
이 변형들은PassType.ForwardBase
에 필요합니다.- –
multi_compile_fwdadd
adds this set of keywords:
(multi_compile_fwdadd_fullshadows는 다음과 같은 키워드 집합을 추가합니다:)
POINT DIRECTIONAL SPOT POINT_COOKIE DIRECTIONAL_COOKIE.
These variants are needed by PassType.ForwardAdd.
(이 변형들은PassType.ForwardBase
에 필요합니다.) - –
multi_compile_fwdadd_fullshadows
adds this set of keywords:
(multi_compile_lightpass
는 다음과 같은 키워드 집합을 추가합니다:)
POINT DIRECTIONAL SPOT POINT_COOKIE DIRECTIONAL_COOKIE SHADOWS_DEPTH SHADOWS_SCREEN SHADOWS_CUBE SHADOWS_SOFT SHADOWS_SHADOWMASK LIGHTMAP_SHADOW_MIXING.
This is the same asmulti_compile_fwdadd
, but this adds the ability for the lights to have real-time shadows.
(이것은multi_compile_fwdadd
와 동일하지만, 실제 그림자를 위한 기능이 추가됩니다.) - –
multi_compile_lightpass
adds this set of keywords:
(multi_compile_shadowcaster
는 다음과 같은 키워드 집합을 추가합니다:)
POINT DIRECTIONAL SPOT POINT_COOKIE DIRECTIONAL_COOKIE SHADOWS_DEPTH SHADOWS_SCREEN SHADOWS_CUBE SHADOWS_SOFT SHADOWS_SHADOWMASK LIGHTMAP_SHADOW_MIXING.
This is effectively a catch-all shortcut for all functionality related to real-time light and shadows, other than Light Probes.
(이것은 실시간 빛과 그림자에 관련된 모든 기능을 포함하는 단축어로, 라이트 프로브를 제외한 모든 것에 해당됩니다.) - –
multi_compile_shadowcaster
adds this set of keywords:
SHADOWS_DEPTH SHADOWS_CUBE.
These variants are needed by PassType.ShadowCaster.
(이 변형들은PassType.ShadowCaster
에 필요합니다.) - –
multi_compile_shadowcollector
adds this set of keywords:
SHADOWS_SPLIT_SPHERES SHADOWS_SINGLE_CASCADE.
It also compiles variants without any of these keywords. These variants are needed for screen-space shadows.
(또한 이러한 키워드 없이 변형들을 컴파일합니다. 이 변형들은 화면 공간 그림자에 필요합니다.) - multi_compile_prepassfinal
adds this set of keywords:
LIGHTMAP_ON DIRLIGHTMAP_COMBINED DYNAMICLIGHTMAP_ON UNITY_HDR_ON SHADOWS_SHADOWMASK LIGHTPROBE_SH.
It also compiles variants without any of these keywords. These variants are needed by PassType.Deferred.
(또한 이러한 키워드 없이 변형들을 컴파일합니다. 이 변형들은PassType.Deferred
에 필요합니다.)
The following shortcuts relate to other settings:
(다음 단축어들은 다른 설정과 관련됩니다: )
- –
multi_compile_particles
adds this keyword relating to the Built-in particle system : SOFTPARTICLES_ON.
(multi_compile_particles
는 기본 제공 파티클 시스템과 관련된 SOFTPARTICLES_ON 키워드를 추가합니다.)
It also compiles variants without this keyword. For more information, see Built-in Particle System.
(또한 이 키워드 없이 변형들을 컴파일합니다. 더 자세한 내용은 Built-in Particle System을 참조하십시오.) - –
multi_compile_fog
adds this set of keywords relating to fog: FOG_LINEAR, FOG_EXP, FOG_EXP2.
(multi_compile_fog
는 안개와 관련된 다음과 같은 키워드 집합을 추가합니다: FOG_LINEAR, FOG_EXP, FOG_EXP2.)
It also compiles variants without any of these keywords. You can control this behavior in the Graphics settings window.
(또한 이러한 키워드 없이 변형들을 컴파일합니다. 이 동작은 그래픽 설정창에서 제어할 수 있습니다.) - –
multi_compile_instancing
adds keywords relating to instancing.
(multi_compile_instancing
은 인스턴싱과 관련된 키워드를 추가합니다.)
If the shader uses procedural instancing, it adds this set of keywords: INSTANCING_ON PROCEDURAL_ON.
(shader가 procedural 인스턴싱을 사용하는 경우, INSTANCING_ON과 PROCEDURAL_ON 키워드 집합을 추가합니다.)
Otherwise, it adds this keyword: INSTANCING_ON. It also compiles variants without any of these keywords.
(그렇지 않으면 INSTANCING_ON 키워드만 추가됩니다. 또한 이러한 키워드 없이 변형들을 컴파일합니다.)
You can control this behavior in the Graphics settings window.
(이 동작은 그래픽 설정 창에서 제어할 수 있습니다.)
Shader semantics (쉐이더 의미론)
When writing HLSL shader programs, input and output variables need to have their “intent” indicated via semantics.
(HLSL shader programs을 작성할 때, 입력 및 출력 변수는 그 “의도”를 의미론을 통해 나타내야 합니다)
This is a standard concept in HLSL shader language; see the Semantics documentation on MSDN for more details.
(이는 HLSL 쉐이더 언어의 표준 개념으로, 자세한 내용은 MSDN의 의미론 문서를 참조하십시오.)
Vertex shader input semantics (정점 쉐이더 입력 의미론)
The main vertex shader function (indicated by the #pragma vertex
directive) needs to have semantics on all the input parameters.
(주요 정점 쉐이더 함수( #pragma vertex
지시어로 표시됨)에는 모든 입력 매개변수에 대한 의미론이 필요합니다.)
These correspond to individual Mesh data elements, like vertex position, normal mesh, and texture coordinates.
(이러한 의미론은 정점 위치, 정점 법선, 텍스처 좌표와 같은 개별 메시 데이터 요소에 해당합니다.)
See vertex program inputs for more details.
(더 자세한 내용은 정점 프로그램 입력을 참조하십시오.)
Here’s an example of a simple vertex shader that takes vertex position and a texture coordinate as an input.
(다음은 정점 위치와 텍스처 좌표를 입력으로 받는 간단한 정점 shader의 예입니다.)
The pixel shader visualizes the texture coordinate as a color.
(픽셀 shader는 텍스처 좌표를 색상으로 시각화합니다.)
Shader "Unlit/Show UVs" { SubShader { Pass { CGPROGRAM #pragma vertex vert #pragma fragment frag struct v2f { float2 uv : TEXCOORD0; float4 pos : SV_POSITION; }; v2f vert ( float4 vertex : POSITION, // vertex position input float2 uv : TEXCOORD0 // first texture coordinate input ) { v2f o; o.pos = UnityObjectToClipPos(vertex); o.uv = uv; return o; } fixed4 frag (v2f i) : SV_Target { return fixed4(i.uv, 0, 0); } ENDCG } } }
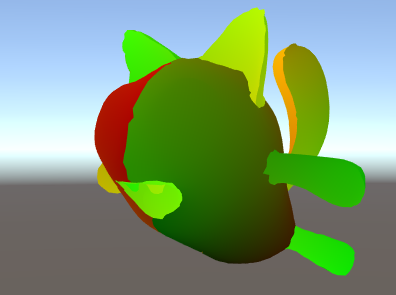
Instead of spelling out all individual inputs one by one, it’s also possible to declare a structure of them, and indicate semantics on each individual member variable of the struct.
(개별 입력을 하나씩 나열하는 대신, 입력들을 구조체로 선언하고 구조체의 각 멤버 변수에 의미론을 지정할 수도 있습니다.)
Fragment shader output semantics (프래그먼트(픽셀) 쉐이더 출력 의미론)
Most often a fragment (pixel) shader outputs a color, and has an SV_Target
semantic. The fragment shader in the example above does exactly that:
(대부분의 경우, 프래그먼트(픽셀) 쉐이더는 색상을 출력하며, SV_Target
의미론을 가집니다. 위의 예제에서 프래그먼트 쉐이더는 정확히 이 작업을 수행합니다.)
struct fragOutput { fixed4 color : SV_Target; }; fragOutput frag (v2f i) { fragOutput o; o.color = fixed4(i.uv, 0, 0); return o; }
Returning structures from the fragment shader is mostly useful for shaders that don’t just return a single color.
(fragment shader에서 구조체를 반환하는 것은 단일 색상 외에 여러 값을 반환해야 하는 shader에 유용합니다.)
Additional semantics supported by the fragment shader outputs are as follows.
(fragment shader 출력에서 지원하는 추가 의미론은 다음과 같습니다.)
SV_TargetN: Multiple render targets
SV_Target1
, SV_Target2
, etc.: These are additional colors written by the shader.
(SV_Target1
, SV_Target2
등은 쉐이더가 추가로 작성하는 색상 값입니다.)
This is used when rendering into more than one render target at once (known as the Multiple Render Targets rendering technique, or MRT). SV_Target0
is the same as SV_Target
.
(이는 한 번에 여러 렌더 타겟에 렌더링할 때 사용됩니다(MRT, Multiple Render Targets 렌더링 기술). SV_Target0
은 SV_Target
과 동일합니다.)
SV_Depth: Pixel shader depth output
Usually the fragment shader doesn’t override the Z buffer value, and a default value is used from the regular triangle rasterization
.
(일반적으로 프래그먼트 shader는 Z 버퍼 값을 덮어쓰지 않으며, 기본 값은 일반적인 삼각형 래스터화에서 사용됩니다.)
However, for some effects it’s useful to output custom Z buffer depth values per pixel.
(그러나 일부 효과에서는 픽셀별로 사용자 정의 Z 버퍼 깊이 값을 출력하는 것이 유용할 수 있습니다.)
Note that on many GPUs this turns off some depth buffer optimizations, so don’t override Z buffer value without a good reason.
많은 GPU에서 이 작업을 수행하면 depth buffer 최적화가 비활성화될 수 있으므로, 특별한 이유 없이 Z 버퍼 값을 덮어쓰지 않는 것이 좋습니다.
The cost incurred by SV_Depth
varies depending on the GPU architecture, but overall it’s fairly similar to the cost of alpha testing (using the built-in clip()
function in HLSL).
(SV_Depth
로 인한 비용은 GPU 아키텍처에 따라 다르지만, 대체로 알파 테스트(내장된 clip()
함수 사용)와 비슷한 비용을 가집니다.)
Render shaders that modify depth after all regular opaque shaders (for example, by using the AlphaTest
rendering queue.
(모든 일반 불투명 쉐이더 후에 깊이를 수정하는 렌더 쉐이더(예: AlphaTest
렌더링 큐를 사용하는 경우)에서 사용됩니다.)
The depth output value needs to be a single float
.
(깊이 출력 값은 단일 float
값이어야 합니다.)
Vertex shader outputs and fragment shader inputs
(Vertex shader 출력과 fragment shader 입력)
A vertex shader needs to output the final clip space position of a vertex, so that the GPU knows where on the screen to rasterize it, and at what depth.
(vertex shader는 꼭지점의 최종 클립 공간 위치를 출력해야 하며, 그래야 GPU가 화면 어디에 그리며 어느 깊이에서 rasterize해야 할지 알 수 있습니다.)
This output needs to have the SV_POSITION
semantic, and be of a float4
type.
(이 출력 값은 SV_POSITION 의미론을 가져야 하며, float4 타입이어야 합니다.)
Any other outputs (“interpolators” or “varyings”) produced by the vertex shader are whatever your particular shader needs.
(vertex shader 생성되는 다른 출력들(“보간자” 또는 “변화값”)은 shader에서 필요한 값들입니다. )
The values output from the vertex shader will be interpolated across the face of the rendered triangles, and the values at each pixel will be passed as inputs to the fragment shader.
(vertex shader에서 출력된 값들은 렌더링된 삼각형 면 전체에 걸쳐 보간되며, 각 픽셀의 값들은 fragment shader로 입력됩니다.)
Many modern GPUs don’t really care what semantics these variables have; however some old systems (most notably, shader model 2 GPUs) did have special rules about the semantics:
(대부분의 최신 GPU는 이 변수들이 어떤 의미론을 가지는지 신경 쓰지 않습니다. 그러나 일부 구형 시스템(특히 shader 모델 2 GPU)은 의미론에 대한 특별한 규칙이 있었습니다.)
- –
TEXCOORD0
,TEXCOORD1
etc are used to indicate arbitrary high precision data such as texture coordinates and positions.
(TEXCOORD0, TEXCOORD1 등은 텍스처 좌표나 위치와 같은 임의의 고정밀 데이터를 나타내는 데 사용됩니다.) - –
COLOR0
andCOLOR1
semantics on vertex outputs and fragment inputs are for low-precision, 0–1 range data (like simple color values).
(vertex 출력과 fragment 입력에서 COLOR0과 COLOR1 의미론은 저정밀도, 0–1 범위의 데이터를 나타내며, 간단한 색상 값과 같은 데이터를 다룹니다.)
For best cross platform support, label vertex outputs and fragment inputs as TEXCOORDn
semantics.
(최적의 플랫폼 호환성을 위해 버텍스 출력과 프래그먼트 입력은 TEXCOORDn 의미론으로 지정하는 것이 좋습니다.)
Interpolator count limits (보간자 개수 제한)
There are limits to how many interpolator variables can be used in total to pass the information from the vertex into the fragment shader.
(vertex에서 fragment shader로 정보를 전달하는 데 사용할 수 있는 보간자 변수의 총 개수에는 제한이 있습니다.)
The limit depends on the platform and GPU, and the general guidelines are:
(이 제한은 플랫폼과 GPU에 따라 다르며, 일반적인 가이드는 다음과 같습니다.)
- – Up to 8 interpolators:
(최대 8개의 보간자: )
Direct3D 11 9.x level (Windows Phone) . Since the interpolator count is limited, but each interpolator can be a 4-component vector, some shaders pack things together to stay within limits. For example, you can pass two texture coordinates in onefloat4
variable (.xy for one coordinate, .zw for the second coordinate).
(Direct3D 11 9.x 수준 (Windows Phone). 보간자 개수가 제한되어 있지만, 각 보간자는 4개의 구성 요소로 이루어진 벡터일 수 있기 때문에, 일부 쉐이더는 제한 내에서 값을 패킹합니다. 예를 들어, 두 개의 텍스처 좌표를 하나의 float4 변수(.xy는 첫 번째 좌표, .zw는 두 번째 좌표)로 전달할 수 있습니다.) - – Up to 10 interpolators: Shader model 3.0 (
#pragma target 3.0
).
(최대 10개의 보간자: 쉐이더 모델 3.0 (#pragma target 3.0).) - – Up to 16 interpolators: OpenGL ES 3.0 (Android), Metal (iOS).
(최대 16개의 보간자: OpenGL ES 3.0 (Android), Metal (iOS).) - – Up to 32 interpolators: Direct3D 10 shader model 4.0 (
#pragma target 4.0
).
(최대 32개의 보간자: Direct3D 10 쉐이더 모델 4.0 (#pragma target 4.0).)
Regardless of your particular target hardware, it’s generally a good idea to use as few interpolators as possible for performance reasons.
(목표 하드웨어와 관계없이 성능을 위해 보간자를 가능한 적게 사용하는 것이 좋습니다.)
Other special semantics (기타 특수 의미론)
Screen space pixel position: VPOS
(스크린 공간 픽셀 위치: VPOS)
A fragment shader can receive position of the pixel being rendered as a special VPOS
semantic.
(fragment 쉐이더는 렌더링 중인 픽셀의 위치를 VPOS라는 특별한 의미론으로 받을 수 있습니다.)
This feature only exists starting with shader model 3.0, so the shader needs to have the #pragma target 3.0
compilation directive.
(이 기능은 shader 모델 3.0부터 존재하므로, shader 는 #pragma target 3.0 컴파일 지시어가 필요합니다.)
On different platforms the underlying type of the screen space position input varies, so for maximum portability use the UNITY_VPOS_TYPE
type for it, which is float4
on most platforms.
(다른 플랫폼에서는 스크린 공간 위치 입력의 기본 타입이 다를 수 있으므로, 최대 호환성을 위해 대부분의 플랫폼에서 float4 타입인 UNITY_VPOS_TYPE을 사용하는 것이 좋습니다.)
Additionally, using the pixel position semantic makes it hard to have both the clip space position (SV_POSITION) and VPOS in the same vertex-to-fragment structure.
(또한 픽셀 위치 의미론을 사용하면 클립 공간 위치(SV_POSITION)와 VPOS를 동일한 vertex-to-fragmen 구조에서 함께 사용하는 것이 어려워집니다.)
Therefore, the vertex shader should output the clip space position as a separate “out” variable. See the example shader below:
(따라서 vertex shader는 클립 공간 위치를 별도의 “출력” 변수로 출력해야 합니다. 아래의 예제 쉐이더를 참조하십시오.)
Shader "Unlit/Screen Position" { Properties { _MainTex ("Texture", 2D) = "white" {} } SubShader { Pass { CGPROGRAM #pragma vertex vert #pragma fragment frag #pragma target 3.0 // note: no SV_POSITION in this struct struct v2f { float2 uv : TEXCOORD0; }; v2f vert ( float4 vertex : POSITION, // vertex position input float2 uv : TEXCOORD0, // texture coordinate input out float4 outpos : SV_POSITION // clip space position output ) { v2f o; o.uv = uv; outpos = UnityObjectToClipPos(vertex); return o; } sampler2D _MainTex; fixed4 frag (v2f i, UNITY_VPOS_TYPE screenPos : VPOS) : SV_Target { // screenPos.xy will contain pixel integer coordinates. // use them to implement a checkerboard pattern that skips rendering // 4x4 blocks of pixels // checker value will be negative for 4x4 blocks of pixels // in a checkerboard pattern screenPos.xy = floor(screenPos.xy * 0.25) * 0.5; float checker = -frac(screenPos.r + screenPos.g); // clip HLSL instruction stops rendering a pixel if value is negative clip(checker); // for pixels that were kept, read the texture and output it fixed4 c = tex2D (_MainTex, i.uv); return c; } ENDCG } } }
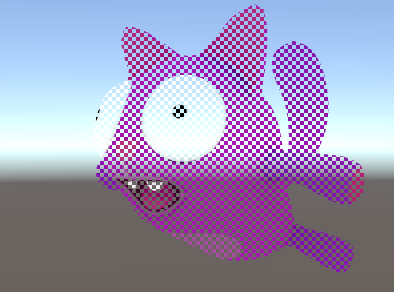
Face orientation: VFACE (면 방향: VFACE)
A fragment shader can receive a variable that indicates whether the rendered surface is facing the camera, or facing away from the camera.
(fragment shader는 렌더링된 표면이 카메라를 향하고 있는지, 아니면 카메라에서 멀어지고 있는지를 나타내는 변수를 받을 수 있습니다.)
This is useful when rendering geometry that should be visible from both sides – often used on leaves and similar thin objects.
(이는 잎사귀와 같은 얇은 객체에서 양면이 모두 보이게 렌더링해야 할 때 유용합니다.)
The VFACE
semantic input variable will contain a positive value for front-facing triangles, and a negative value for back-facing ones.
(VFACE 의미론 입력 변수는 앞면을 향하는 삼각형에는 양의 값을, 뒷면을 향하는 삼각형에는 음의 값을 포함합니다.)
This feature only exists from shader model 3.0 onwards, so the shader needs to have the #pragma target 3.0
compilation directive.
(이 기능은 쉐이더 모델 3.0부터 존재하므로, 쉐이더는 #pragma target 3.0 컴파일 지시어가 필요합니다.)
Shader "Unlit/Face Orientation" { Properties { _ColorFront ("Front Color", Color) = (1,0.7,0.7,1) _ColorBack ("Back Color", Color) = (0.7,1,0.7,1) } SubShader { Pass { Cull Off // turn off backface culling CGPROGRAM #pragma vertex vert #pragma fragment frag #pragma target 3.0 float4 vert (float4 vertex : POSITION) : SV_POSITION { return UnityObjectToClipPos(vertex); } fixed4 _ColorFront; fixed4 _ColorBack; fixed4 frag (fixed facing : VFACE) : SV_Target { // VFACE input positive for frontbaces, // negative for backfaces. Output one // of the two colors depending on that. return facing > 0 ? _ColorFront : _ColorBack; } ENDCG } } }
The shader above uses the Cull state to disable back-face culling (by default back-facing triangles aren’t rendered at all).
(위의 shader 는 Cull 상태를 사용하여 후면 컬링을 비활성화합니다. 기본적으로 후면 삼각형은 전혀 렌더링되지 않습니다.)
Here is the shader applied to a bunch of Quad meshes, rotated at different orientations:
(다양한 방향으로 회전된 여러 개의 쿼드 메시들에 적용된 쉐이더는 다음과 같습니다:)
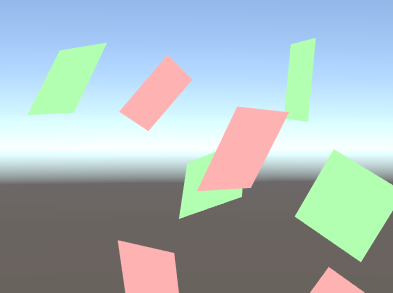
Vertex ID: SV_VertexID (Vertex ID: SV_VertexID)
A vertex shader can receive a variable that has the “vertex number” as an unsigned integer.
(정점 쉐이더는 ‘정점 번호’를 부호 없는 정수로 받을 수 있습니다.)
This is mostly useful when you want to fetch additional per-vertex data from textures or ComputeBuffers.
(이는 주로 텍스처나 ComputeBuffers에서 추가적인 per-vertex data를 가져올 때 유용합니다.)
This feature only exists from DX10 (shader model 4.0) and GLCore / OpenGL ES 3, so the shader needs to have the #pragma target 3.5
compilation directive.
(이 기능은 DX10(쉐이더 모델 4.0)과 GLCore/OpenGL ES 3부터 존재하므로, 쉐이더는 #pragma target 3.5 컴파일 지시어를 포함해야 합니다.)
Shader "Unlit/VertexID" { SubShader { Pass { CGPROGRAM #pragma vertex vert #pragma fragment frag #pragma target 3.5 struct v2f { fixed4 color : TEXCOORD0; float4 pos : SV_POSITION; }; v2f vert ( float4 vertex : POSITION, // vertex position input uint vid : SV_VertexID // vertex ID, needs to be uint ) { v2f o; o.pos = UnityObjectToClipPos(vertex); // output funky colors based on vertex ID float f = (float)vid; o.color = half4(sin(f/10),sin(f/100),sin(f/1000),0) * 0.5 + 0.5; return o; } fixed4 frag (v2f i) : SV_Target { return i.color; } ENDCG } } }
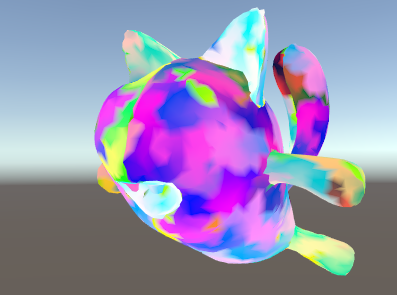
Accessing shader properties in Cg/HLSL
(Cg/HLSL에서 셰이더 속성 접근)
Shader declares Material properties in a Properties block.
(Shader는 Properties 블록에서 머티리얼 속성을 선언합니다. )
If you want to access some of those properties in a shader program, you need to declare a Cg/HLSL variable with the same name and a matching type.|
(shader program에서 해당 속성 중 일부에 접근하려면, 동일한 이름과 일치하는 타입을 가진 Cg/HLSL 변수를 선언해야 합니다. )
For example these shader properties:
(예를 들어, 이러한 shader 속성들 : )
_MyColor ("Some Color", Color) = (1,1,1,1) _MyVector ("Some Vector", Vector) = (0,0,0,0) _MyFloat ("My float", Float) = 0.5 _MyTexture ("Texture", 2D) = "white" {} _MyCubemap ("Cubemap", CUBE) = "" {}
would be declared for access in Cg/HLSL code as:
(Cg/HLSL 코드에서 다음과 같이 선언됩니다: )
fixed4 _MyColor; // low precision type is usually enough for colors (색상에는 일반적으로 낮은 정밀도의 타입이 충분) float4 _MyVector; float _MyFloat; sampler2D _MyTexture; samplerCUBE _MyCubemap;
Cg/HLSL can also accept uniform keyword, but it is not necessary:
(Cg/HLSL에서는 uniform 키워드를 사용할 수 있지만, 반드시 필요한 것은 아닙니다: )
uniform float4 _MyColor;
Property types in ShaderLab map to Cg/HLSL variable types this way:
- – Color and Vector properties map to float4, half4 or fixed4 variables.
(Color와 Vector 속성은 float4, half4 또는 fixed4 변수에 매핑됩니다. ) - – Range and Float properties map to float, half or fixed variables.
(Range와 Float 속성은 float, half 또는 fixed 변수에 매핑됩니다.) - – Texture properties map to sampler2D variables for regular (2D) textures; Cubemaps map to samplerCUBE; and 3D textures map to sampler3D.
(텍스처 속성은 일반 (2D) textures의 경우 sampler2D 변수에, Cubemaps의 경우 samplerCUBE, 3D 텍스처는 sampler3D에 매핑됩니다. )
How property values are provided to shaders (속성 값이 shader에 제공되는 방법)
Shader property values are found and provided to shaders from these places:
(셰이더 속성 값은 다음과 같은 경로로 셰이더에 전달됩니다: )
- – Per-Renderer values set in MaterialPropertyBlock. This is typically “per-instance” data (e.g. customized tint color for a lot of objects that all share the same material).
MaterialPropertyBlock에 설정된 렌더러별 값. 이는 일반적으로 “인스턴스별” 데이터입니다 (예: 동일한 머티리얼을 공유하는 여러 객체에 대한 맞춤형 색상). - – Values set in the Material that’s used on the rendered object.
(렌더링된 객체에 사용된 머티리얼에 설정된 값. ) - – Global shader properties, set either by Unity rendering code itself (see built-in shader variables), or from your own scripts (e.g. Shader.SetGlobalTexture).
전역 셰이더 속성은 Unity의 렌더링 코드에서 설정되거나(내장 셰이더 변수 참조), 스크립트에서 직접 설정됩니다(예: Shader.SetGlobalTexture).
The order of precedence is like above: per-instance data overrides everything; then Material data is used; and finally if shader property does not exist in these two places then global property value is used.
(우선순위는 위와 같습니다: 인스턴스별 데이터가 모든 것을 덮어쓰며, 그다음으로 머티리얼 데이터가 사용됩니다. 이 두 곳에 shader 속성이 없으면 전역 속성 값이 사용됩니다.)
Finally, if there’s no shader property value defined anywhere, then “default” (zero for floats, black for colors, empty white texture for textures) value will be provided.
(마지막으로, 어느 곳에도 shader 속성이 정의되어 있지 않으면 “기본값”(float의 경우 0, 색상의 경우 검정, 텍스처의 경우 빈 흰색 텍스처)이 제공됩니다. )
Serialized and Runtime Material properties
(직렬화된 머티리얼 속성과 런타임 머티리얼 속성)
Materials can contain both serialized and runtime-set property values.
(Materials은 직렬화된 값과 런타임에서 설정된 속성 값을 모두 포함할 수 있습니다. )
Serialized data is all the properties defined in shader’s Properties block.
(직렬화된 데이터는 셰이더의 Properties 블록에 정의된 모든 Properties입니다.)
Typically these are values that need to be stored in the material, and are tweakable by the user in Material Inspector.
(일반적으로 이러한 값들은 material에 저장되어야 하며, Material Inspector에서 사용자가 조정할 수 있습니다.)
A material can also have some properties that are used by the shader, but not declared in shader’s Properties block.
material 에는 shader에서 사용되지만 shader의 Properties 블록에 선언되지 않은 속성도 있을 수 있습니다.
Typically this is for properties that are set from script code at runtime, e.g. via Material.SetColor.
(이러한 속성은 일반적으로 런타임에서 스크립트 코드로 설정됩니다(예: Material.SetColor를 통해).)
Note that matrices and arrays can only exist as non-serialized runtime properties (since there’s no way to define them in Properties block).
(행렬과 배열은 Properties 블록에서 정의할 수 없기 때문에 직렬화되지 않은 런타임 속성으로만 존재할 수 있다는 점에 유의해야 합니다.)
Special Texture properties (특수 텍스처 속성)
For each texture that is setup as a shader/material property, Unity also sets up some extra information in additional vector properties.
(shader/material 속성으로 설정된 각 텍스처에 대해 Unity는 추가 벡터 속성에 추가 정보를 설정합니다.)
Texture tiling & offset (텍스처 타일링 및 오프셋)
Materials often have Tiling and Offset fields for their texture properties.
(Materials은 종종 텍스처 속성에 대해 타일링 및 오프셋 필드를 가집니다.)
This information is passed into shaders in a float4 {TextureName}_ST
property:
(이 정보는 float4 {TextureName}_ST 속성으로 shaders에 전달됩니다.)
- –
x
contains X tiling value (x는 X 타일링 값을 포함합니다) - –
y
contains Y tiling value (y는 Y 타일링 값을 포함합니다) - –
z
contains X offset value (z는 X 오프셋 값을 포함합니다) - –
w
contains Y offset value (w는 Y 오프셋 값을 포함합니다)
For example, if a shader contains texture named _MainTex
, the tiling information will be in a _MainTex_ST
vector.
(예를 들어, shader에 _MainTex라는 텍스처가 있을 경우, 타일링 정보는 _MainTex_ST 벡터에 포함됩니다.)
Texture size (텍스처 크기)
{TextureName}_TexelSize
– a float4 property contains texture size information:
({TextureName}_TexelSize – float4 속성은 텍스처 크기 정보를 포함합니다 : )
- –
x
contains 1.0/width (x는 1.0/너비 값을 포함합니다) - –
y
contains 1.0/height (y는 1.0/높이 값을 포함합니다) - –
z
contains width (z는 너비를 포함합니다) - –
w
contains height (w는 높이를 포함합니다)
Texture HDR parameters (Texture HDR 매개변수)
{TextureName}_HDR
– a float4 property with information on how to decode a potentially HDR (e.g. RGBM-encoded) texture depending on the color space used.
({TextureName}_HDR – 사용된 색 공간에 따라 HDR (예: RGBM 인코딩된) 텍스처를 디코딩하는 방법에 대한 정보를 포함하는 float4 속성입니다.)
See DecodeHDR
function in UnityCG.cginc shader include file.
(자세한 내용은UnityCG.cginc shader 포함 파일의 DecodeHDR 함수를 참조하세요.)
Color spaces and color/vector shader data (색 공간 및 색상/벡터 shader 데이터)
When using Linear color space, all material color properties are supplied as sRGB colors, but are converted into linear values when passed into shaders.
( Linear color space을 사용할 때, 모든 Material 색상 속성은 sRGB 색상으로 제공되며, shader로 전달될 때 선형 값으로 변환됩니다.)
For example, if your Properties shader block contains a Color
property called “MyColor“, then the corresponding ”MyColor” HLSL variable will get the linear color value.
(예를 들어, Properties 셰이더 블록에 “MyColor”라는 Color 속성이 있으면, 해당 “MyColor” HLSL 변수는 선형 색상 값을 받게 됩니다.)
For properties that are marked as Float
or Vector
type, no color space conversions are done by default; it is assumed that they contain non-color data.
(Float 또는 Vector 타입으로 표시된 속성의 경우 기본적으로 색 공간 변환이 수행되지 않으며, 이들은 색상 데이터가 아닌 것으로 간주됩니다.)
It is possible to add [Gamma]
attribute for float/vector properties to indicate that they are specified in sRGB space, just like colors (see Properties).
(색상과 마찬가지로 float/vector 속성이 sRGB 공간에서 지정된다는 것을 나타내기 위해 [Gamma] 속성을 추가할 수 있습니다 (Properties 참조).)
See Also (참고)
Providing vertex data to vertex programs (버텍스 프로그램에 버텍스 데이터 제공)
https://docs.unity3d.com/2023.2/Documentation/Manual/SL-VertexProgramInputs.html
For Cg/HLSL vertex programs, the Mesh vertex data is passed as inputs to the vertex shader function.
Cg/HLSL vertex programs에서는 Mesh 버텍스 데이터가 vertex shader 함수의 입력으로 전달됩니다.
Each input needs to have a semantic specified for it: for example, POSITION
input is the vertex position, and NORMAL
is the vertex normal.
(각 입력에는 의미(semantic)가 지정되어야 합니다. 예를 들어, POSITION 입력은 vertex 위치이고, NORMAL은 vertex 노멀입니다.)
Often, vertex data inputs are declared in a structure, instead of listing them one by one.
(종종 vertex 데이터 입력은 하나씩 나열하는 대신 구조체로 선언됩니다.)
Several commonly used vertex structures are defined in UnityCG.cginc include file, and in most cases it’s enough just to use those. The structures are:
(UnityCG.cginc include file에 여러 가지 일반적으로 사용되는 버텍스 구조체가 정의되어 있으며, 대부분의 경우 이를 사용하는 것 만으로충분합니다. 이 구조체들은 다음과 같습니다 : )
- –
appdata_base
: position, normal and one texture coordinate. (위치, 노멀 및 하나의 텍스처 좌표.) - –
appdata_tan
: position, tangent, normal and one texture coordinate. (위치, 탄젠트, 노멀 및 하나의 텍스처 좌표.) - –
appdata_full
: position, tangent, normal, four texture coordinates and color. (위치, 탄젠트, 노멀, 네 개의 텍스처 좌표 및 색상.)
Example: This shader colors the mesh based on its normals, and uses appdata_base
as vertex program input:
(예시: 이 shader는 노멀에 기반하여 메시에 색상을 입히며, appdata_base를 버텍스 프로그램 입력으로 사용합니다 : )
Shader "VertexInputSimple" { SubShader { Pass { CGPROGRAM #pragma vertex vert #pragma fragment frag #include "UnityCG.cginc" struct v2f { float4 pos : SV_POSITION; fixed4 color : COLOR; }; v2f vert (appdata_base v) { v2f o; o.pos = UnityObjectToClipPos(v.vertex); o.color.xyz = v.normal * 0.5 + 0.5; o.color.w = 1.0; return o; } fixed4 frag (v2f i) : SV_Target { return i.color; } ENDCG } } }
To access different vertex data, you need to declare the vertex structure yourself, or add input parameters to the vertex shader.
(다른 버텍스 데이터에 접근하려면, 직접 버텍스 구조체를 선언하거나 버텍스 shader에 입력 파라미터를 추가해야 합니다.)
Vertex data is identified by Cg/HLSL semantics, and must be from the following list:
(버텍스 데이터는 Cg/HLSL 의미(semantics)에 의해 식별되며, 다음 목록 중 하나여야 합니다 : )
- –
POSITION
is the vertex position, typically afloat3
orfloat4
. (POSITION은 버텍스 위치로, 일반적으로 float3 또는 float4입니다.) - –
NORMAL
is the vertex normal, typically afloat3
. (NORMAL은 버텍스 노멀로, 일반적으로 float3입니다.) - –
TEXCOORD0
is the first UV coordinate, typicallyfloat2
,float3
orfloat4
. (TEXCOORD0은 첫 번째 UV 좌표로, 일반적으로 float2, float3 또는 float4입니다.) - –
TEXCOORD1
,TEXCOORD2
andTEXCOORD3
are the 2nd, 3rd and 4th UV coordinates, respectively. (TEXCOORD1, TEXCOORD2 및 TEXCOORD3는 각각 두 번째, 세 번째, 네 번째 UV 좌표입니다.) - –
TANGENT
is the tangent vector (used for normal mapping), typically afloat4
. (TANGENT는 탄젠트 벡터(노멀 맵핑에 사용되며), 일반적으로 float4입니다.) - –
COLOR
is the per-vertex color, typically afloat4
. (COLOR는 버텍스당 색상으로, 일반적으로 float4입니다.)
When the mesh data contains fewer components than are needed by the vertex shader input, the rest are filled with zeroes, except for the .w
component which defaults to 1.
(메시 데이터가 vertex shader 입력에서 요구하는 구성 요소보다 적은 경우, 나머지는 0으로 채워지며, .w 구성 요소는 기본적으로 1이 됩니다.)
For example, mesh texture coordinates are often 2D vectors with just x and y components.
(예를 들어, 메시의 텍스처 좌표는 종종 x와 y 구성 요소만 있는 2D 벡터입니다.)
If a vertex shader declares a float4
input with TEXCOORD0
semantic, the value received by the vertex shader will contain (x,y,0,1).
(만약 vertex shader가 TEXCOORD0 의미로 float4 입력을 선언하면, vertex shader가 받는 값은 (x, y, 0, 1)이 됩니다.)
For examples of using these techniques to visualize vertex data in the Built-in Render Pipeline, see Visualizing vertex data.
(이 기술을 사용하여 기본 렌더 파이프라인에서 버텍스 데이터를 시각화하는 예시는 Visualizing vertex data“를 참조하십시오.)
Built-in shader include files
https://docs.unity3d.com/2023.2/Documentation/Manual/SL-BuiltinIncludes.html
Unity contains several files that can be used by your shader programs to bring in predefined variables and helper functions.
(Unity에는 미리 정의된 변수와 헬퍼 함수들을 shader programs에서 사용할 수 있도록 하는 여러 파일이 포함되어 있습니다.)
This is done by the standard #include
directive, e.g.:
(이는 표준 #include 지시어를 사용하여 실행됩니다. 예를 들어 : )
CGPROGRAM // ... #include "UnityCG.cginc" // ... ENDCG
Shader include files in Unity are with .cginc
extension, and the built-in ones are:
(Unity의 셰이더 인클루드 파일은 .cginc 확장자를 가지며, 내장된 파일들은 다음과 같습니다:)
- –
HLSLSupport.cginc
– (automatically included) Helper macros and definitions for cross-platform shader compilation.
(자동으로 포함) 크로스 플랫폼 shader 컴파일을 위한 헬퍼 매크로 및 정의.) - –
UnityShaderVariables.cginc
– (automatically included) Commonly used global variables.
((자동으로 포함) 일반적으로 사용되는 전역 변수.) - –
UnityCG.cginc
– commonly used helper functions.
(일반적으로 사용되는 helper functions.) - –
AutoLight.cginc
– lighting & shadowing functionality, e.g. surface shaders use this file internally.
(조명 및 그림자 기능, 예를 들어 surface shaders는 이 파일을 내부적으로 사용합니다.) - –
Lighting.cginc
– standard surface shader lighting models; automatically included when you’re writing surface shaders.
(표준 서피스 셰이더 조명 모델; surface shader를 작성할 때 자동으로 포함됩니다.) - –
TerrainEngine.cginc
– helper functions for Terrain & Vegetation shaders.
(지형 및 식물 shader 헬퍼 함수.)
These files are found inside Unity application ({unity install path}/Data/CGIncludes/UnityCG.cginc on Windows, /Applications/Unity/Unity.app/Contents/CGIncludes/UnityCG.cginc on Mac), if you want to take a look at what exactly is done in any of the helper code.
(이 파일들은 Unity 애플리케이션 내부에서 찾을 수 있습니다 ({유니티 설치 경로}/Data/CGIncludes/UnityCG.cginc, Windows의 경우 /Applications/Unity/Unity.app/Contents/CGIncludes/UnityCG.cginc, Mac의 경우). 필요한 경우 헬퍼 코드에서 실제로 수행되는 작업을 확인할 수 있습니다.)
HLSLSupport.cginc
This file is automatically included when compiling CGPROGRAM shaders (but not included for HLSLPROGRAM ones).
(이 파일은 CGPROGRAM 셰이더를 컴파일할 때 자동으로 포함되며, HLSLPROGRAM에는 포함되지 않습니다.)
It declares various preprocessor macros to aid in multi-platform shader development.
(멀티 플랫폼 shader 개발을 돕기 위한 여러 preprocessor macros을 선언합니다.)
UnityShaderVariables.cginc
This file is automatically included when compiling CGPROGRAM shaders (but not included for HLSLPROGRAM ones).
(이 파일은 CGPROGRAM shader를 컴파일할 때 자동으로 포함되며, HLSLPROGRAM에는 포함되지 않습니다.)
It declares various built-in global variables that are commonly used in shaders.
(shader에서 일반적으로 사용되는 다양한 내장 전역 변수를 선언합니다.)
UnityCG.cginc
This file is often included in Shader objects.
(이 파일은 Shader objects에 자주 포함됩니다.)
It declares many built-in helper functions and data structures.
(많은 built-in helper functions와 데이터 구조를 선언합니다.)
Data structures in UnityCG.cginc (UnityCG.cginc의 데이터 구조)
- struct
appdata_base
: vertex shader input with position, normal, one texture coordinate. (위치, 법선, 하나의 텍스처 좌표를 가진 버텍스 셰이더 입력..) - struct
appdata_tan
: vertex shader input with position, normal, tangent, one texture coordinate. (위치, 법선, 탄젠트, 하나의 텍스처 좌표를 가진 버텍스 셰이더 입력.) - struct
appdata_full
: vertex shader input with position, normal, tangent, vertex color and two texture coordinates. (위치, 법선, 탄젠트, 버텍스 색상 및 두 개의 텍스처 좌표를 가진 버텍스 셰이더 입력.) - struct
appdata_img
: vertex shader input with position and one texture coordinate. (위치와 하나의 텍스처 좌표를 가진 버텍스 셰이더 입력.)